What is the Basic Structure of a Program?
Table of Contents
Toggle1. Introduction to Programming
What is Programming?
Programming is the art of writing code that instructs a computer to perform specific tasks. This involves using programming languages like Python, Java, or C++ to create applications, solve problems, or automate processes. At its core, programming bridges the gap between human ideas and machine execution, enabling us to leverage technology for various purposes.
Importance of Understanding Program Structure
The structure of a program serves as its blueprint. Just like architects need a solid plan to build a sturdy house, programmers must follow a systematic structure to create functional and efficient software. A well-structured program enhances readability, reduces bugs, and makes it easier to maintain or scale. It also ensures that the logic of the program aligns seamlessly with its intended purpose.
Unlock Your Business Intelligence Potential with Power BI!
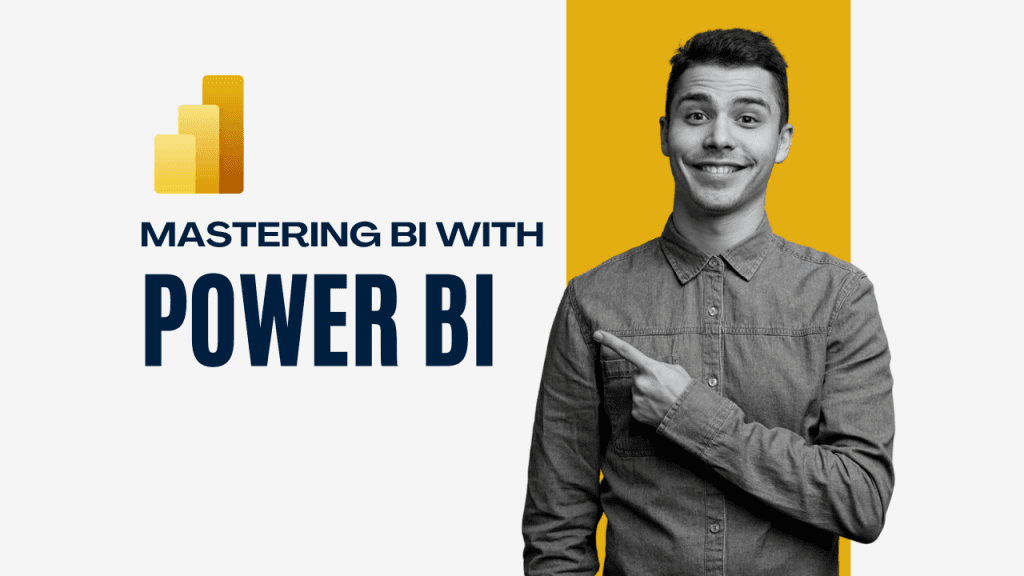
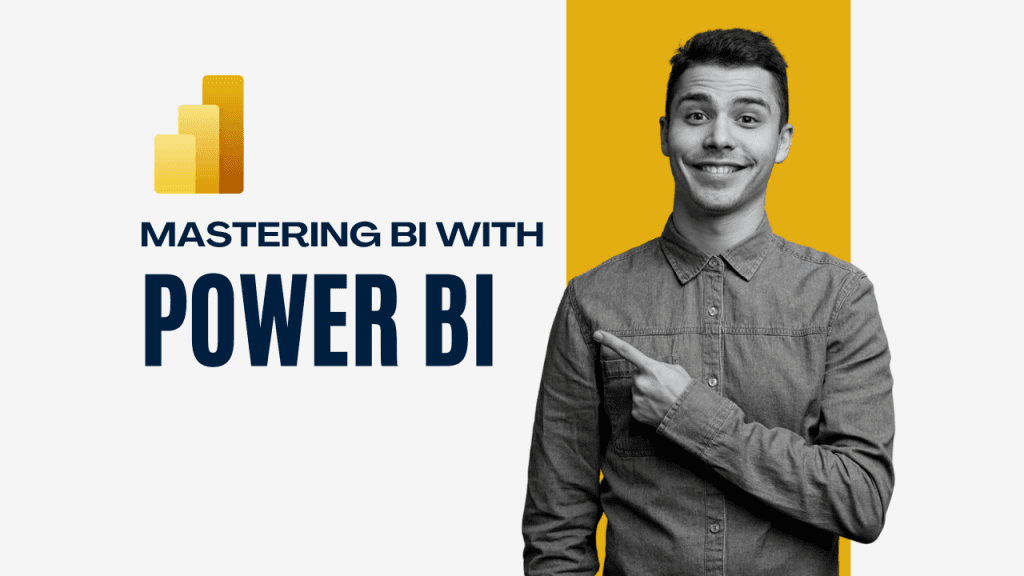
Unlock Your Business Intelligence Potential with Power BI!
2. Components of a Program
Input Section
The input section is where data is gathered to process within the program. Inputs can come from users, files, sensors, or other programs. For instance, in a calculator program, the numbers and operations entered by the user act as the input.
Processing Section
This is the core of the program, where the input data is manipulated or analyzed to produce meaningful results. It involves calculations, data sorting, or logic-based decisions. For example, in a temperature converter, the formula to convert Celsius to Fahrenheit occurs here.
Output Section
The output section delivers the results of the processing to the user or another system. It could be in the form of visual feedback, written reports, or data transmission. A common example is displaying a result on a screen or printing a receipt.
3. Program Skeleton and Syntax
General Syntax of a Program
Every program follows a specific syntax based on the programming language used. This includes defining functions, variables, and logical statements in a structured way. Syntax errors can prevent a program from running and are one of the first challenges new programmers face.
Main Function or Entry Point
Most programming languages designate a main function or entry point where the execution begins. For instance, in C++, the int main()
function acts as the starting line for the program. This ensures a predictable flow of execution and simplifies debugging.
4. Variables and Data Types
What are Variables?
Variables are placeholders used to store data in a program. They act as containers that can hold different values, which the program can manipulate or reference during execution. For example, a variable named temperature
might store the value 25
.
Common Data Types in Programming
Data types define the kind of data a variable can hold. Common data types include:
- Integer (int): Whole numbers like 10 or -5.
- Floating-point (float): Numbers with decimal points like 3.14.
- Character (char): Single letters or symbols, e.g., ‘A’.
- String: A sequence of characters, like “Hello”.
- Boolean: True or false values, often used in decision-making processes.
5. Control Structures
Conditional Statements
Conditional statements guide the flow of a program based on conditions. For example:
if temperature > 30:
print("It's hot outside!")
else:
print("It's a pleasant day.")
These statements help programs make decisions dynamically.
Loops and Iteration
Loops are used for repeating actions until a condition is met. Common loop types include:
- For Loop: Iterates a fixed number of times.
- While Loop: Continues until a condition becomes false.
Example:
for i in range(5):
print("Iteration", i)
Unlock Your Business Intelligence Potential with Power BI!
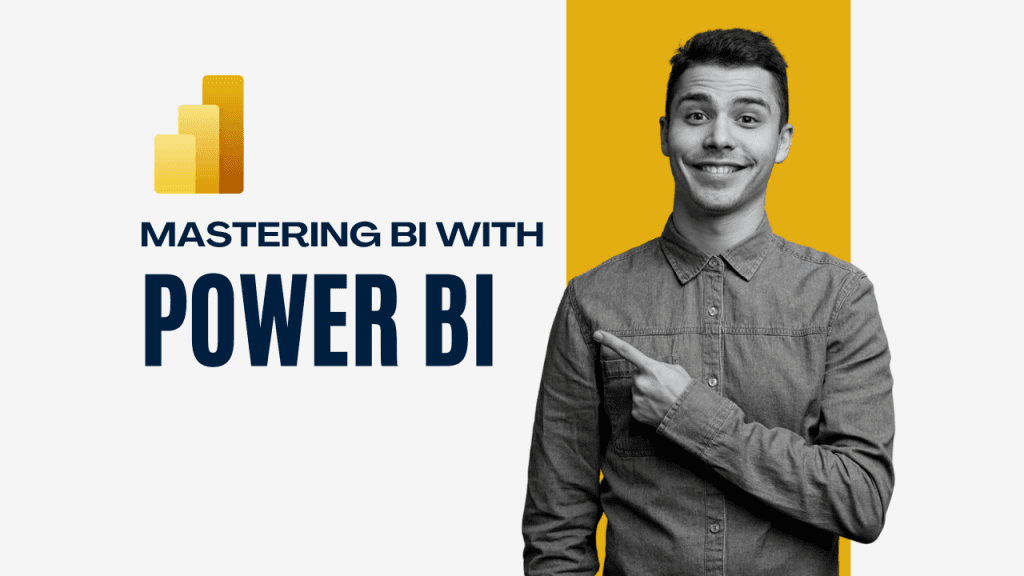
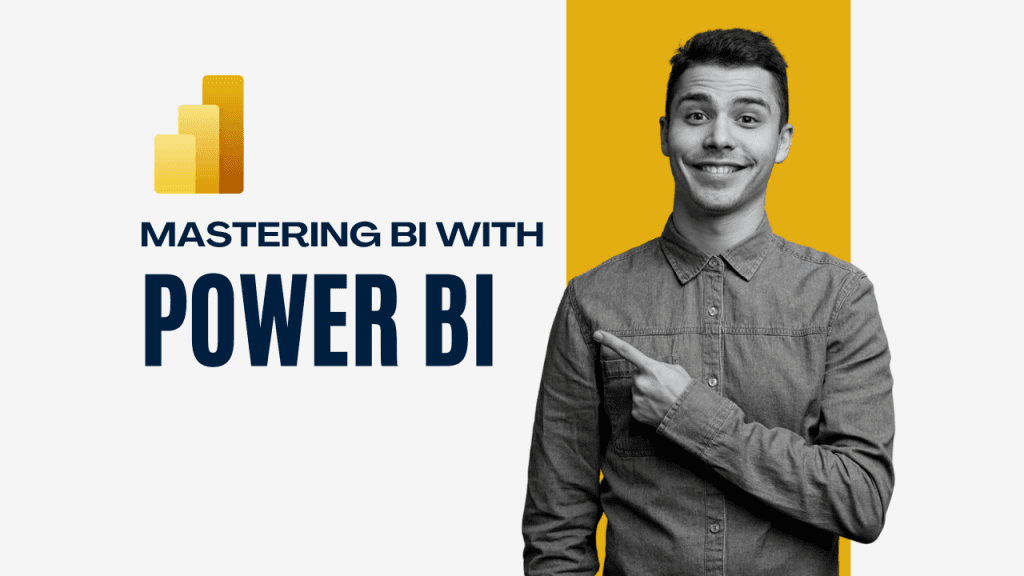
Unlock Your Business Intelligence Potential with Power BI!
6. Functions and Subroutines
Defining Functions
Functions are reusable blocks of code that perform specific tasks. They help break down complex problems into smaller, manageable parts.
Example:
def greet(name):
print("Hello,", name)
Benefits of Modular Programming
Using functions promotes modularity, making programs easier to read, debug, and maintain. This approach is essential for team-based software development, where multiple programmers collaborate.
7. Error Handling and Debugging
Types of Errors in Programming
- Syntax Errors: Caused by incorrect code structure.
- Runtime Errors: Occur while the program is running, such as dividing by zero.
- Logical Errors: The program runs but produces incorrect results due to flawed logic.
Debugging Techniques
- Using print statements to trace variable values.
- Employing debugging tools in IDEs like PyCharm or Visual Studio.
- Writing unit tests to validate individual code segments.
8. Libraries and Frameworks
Role of Libraries
Libraries provide pre-written code that simplifies tasks. For example, Python’s math
library includes functions for complex calculations.
Introduction to Frameworks
Frameworks like Django (for web development) or TensorFlow (for machine learning) provide a structured environment to build specific types of programs faster and more efficiently.
FAQs: Understanding Program Structures
1. What are the main components of a program?
A program typically has three main components: input, processing, and output.
2. Why is program structure important?
A clear structure makes programs easier to read, debug, and scale.
3. How do comments improve program readability?
Comments provide explanations within the code, helping developers understand logic and intentions.
4. What’s the difference between syntax errors and runtime errors?
Syntax errors occur due to incorrect code structure, while runtime errors happen during program execution.
5. How can I learn to write better programs?
Practice regularly, study examples, and follow coding best practices.
6. What role does modular programming play in large-scale software development?
Modular programming divides complex tasks into smaller parts, simplifying development and collaboration.
Unlock Your Business Intelligence Potential with Power BI!
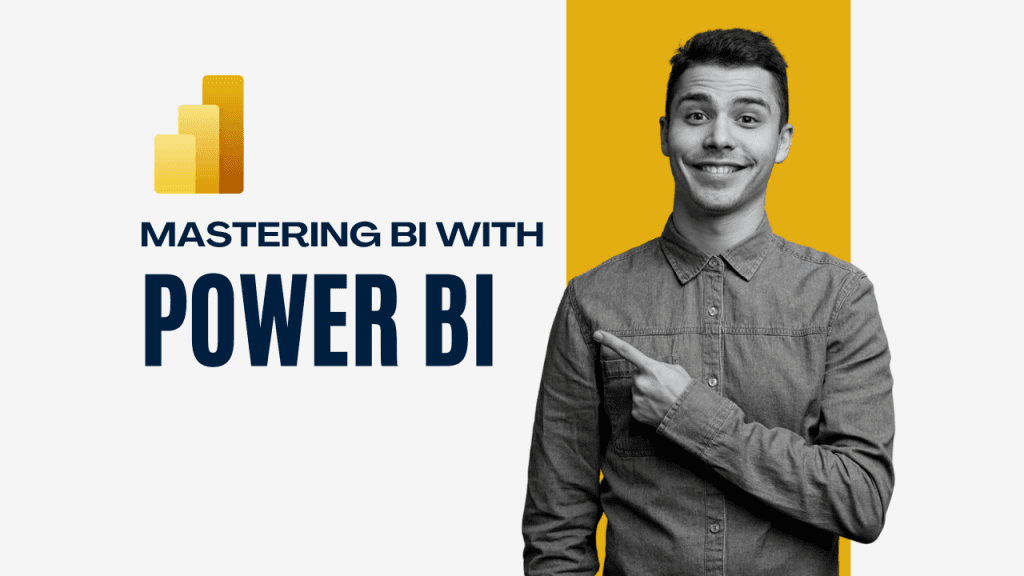
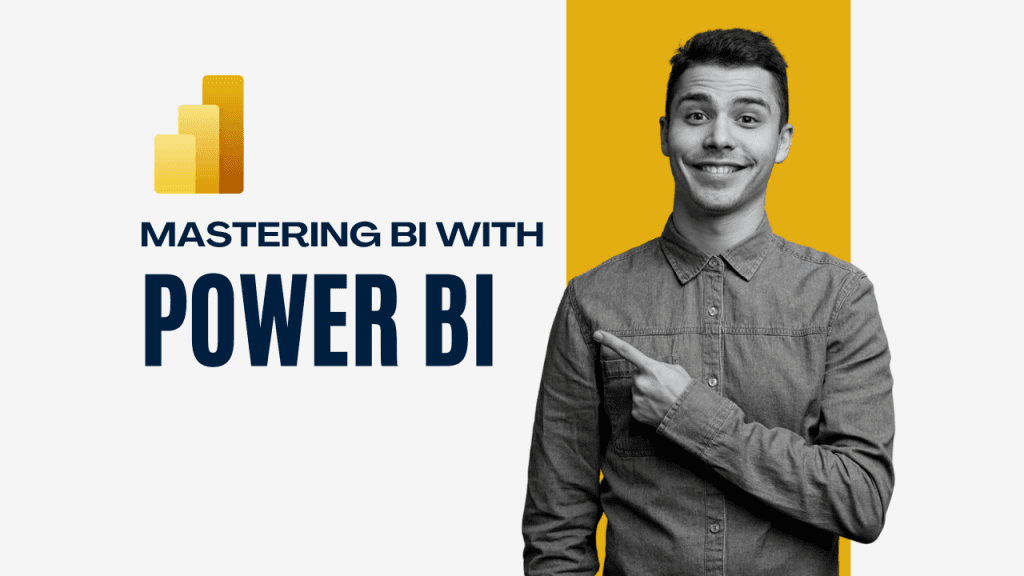