Java programming is one of the most popular programming languages used worldwide due to its platform independence, robustness, and vast library support. Understanding the structure of a Java program is crucial for beginners and those preparing for Java interview questions. In this article, we will explain the structure of a Java program in detail and provide insights to help you ace interviews, especially for topics like Java 8 programming interview questions.
Table of Contents
ToggleUnlock Your Business Intelligence Potential with Power BI!
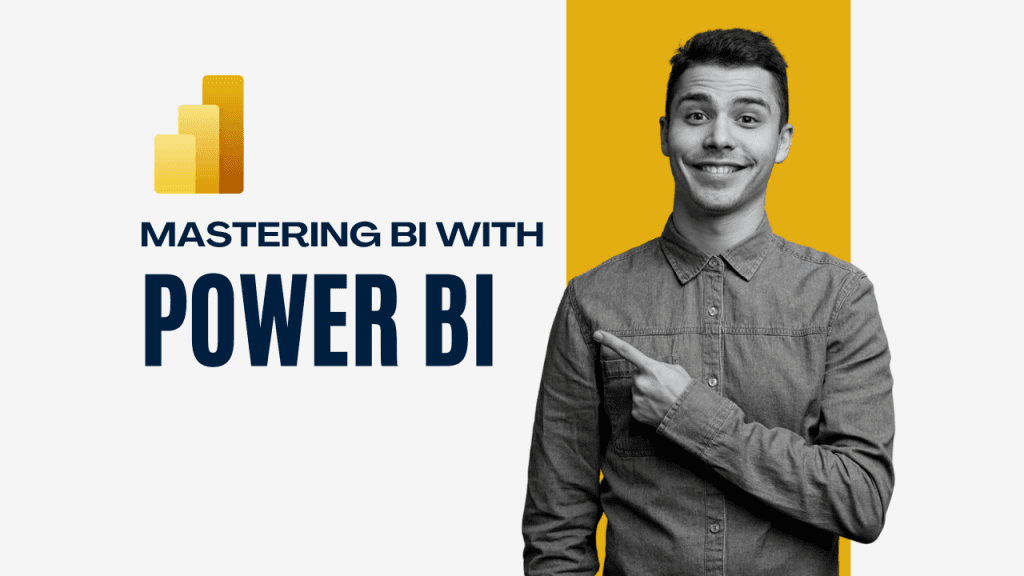
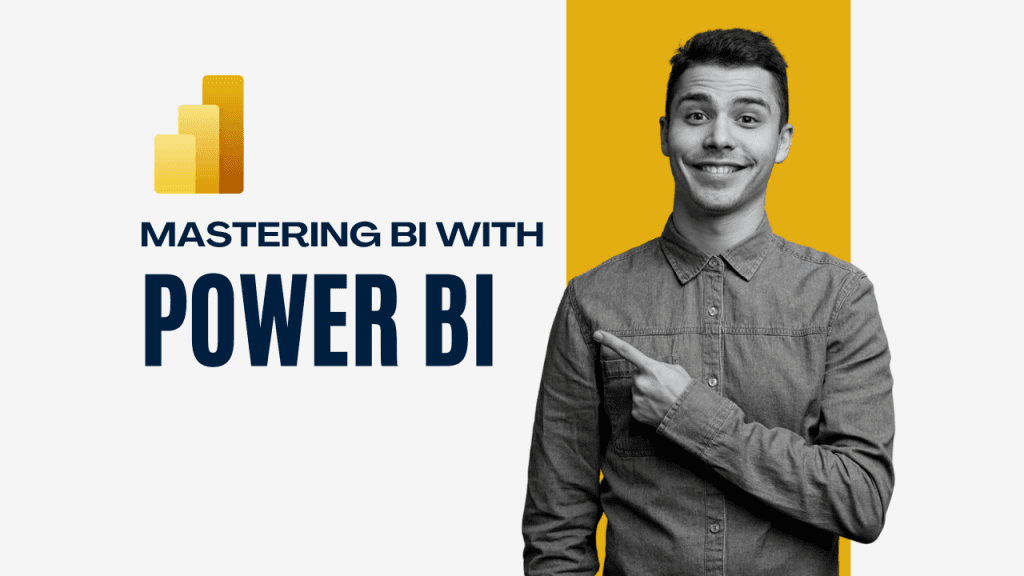
Unlock Your Business Intelligence Potential with Power BI!
The Basic Structure of a Java Program
The structure of a Java program follows a logical and organized format, ensuring readability and maintainability. Below are the key components of a Java program structure:
1. Package Declaration
Every Java program starts with an optional package declaration, which organizes classes into namespaces.
package com.example.myapp;
This declaration helps in avoiding name conflicts and organizing files. Though optional, it’s highly recommended for large projects.
2. Import Statements
The next part is the import statements, used to include built-in or custom classes in your program.
import java.util.Scanner;
By importing necessary libraries, you can access predefined functions and classes, saving time in coding.
3. Class Declaration
The core part of the structure of a Java program is the class declaration. Each Java program must contain at least one class.
public class HelloWorld {
// Class body
}
Here, HelloWorld
is the class name, and the public
access modifier makes it accessible across the projec
4. Main Method
The main method is the entry point of any Java program. It controls the flow of the program and executes the code.
public static void main(String[] args) {
System.out.println(“Hello, World!”);
}
The main method has the following parts:
public
: Makes the method accessible from anywhere.static
: Allows the method to run without instantiating a class.void
: Specifies that the method does not return a value.String[] args
: Accepts command-line arguments.
5. Variables and Methods
Within the class, you define variables and methods to store data and implement functionality.
public static void main(String[] args) {
System.out.println(“Hello, World!”);
}
int number = 10; // Variable
public void displayMessage() {
System.out.println(“Message Displayed”);
}
These are key elements that make the class functional and dynamic.
Unlock Your Business Intelligence Potential with Power BI!
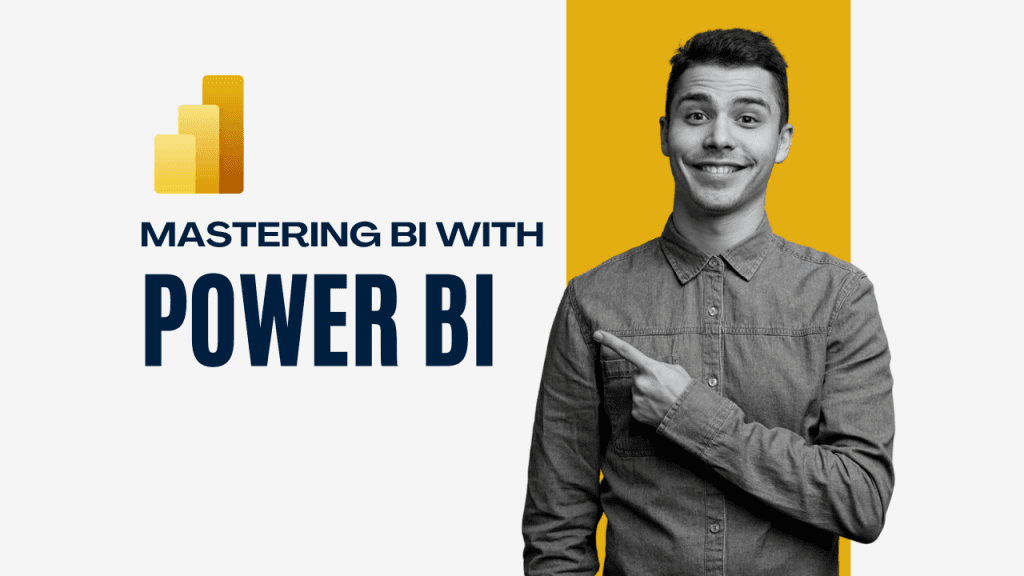
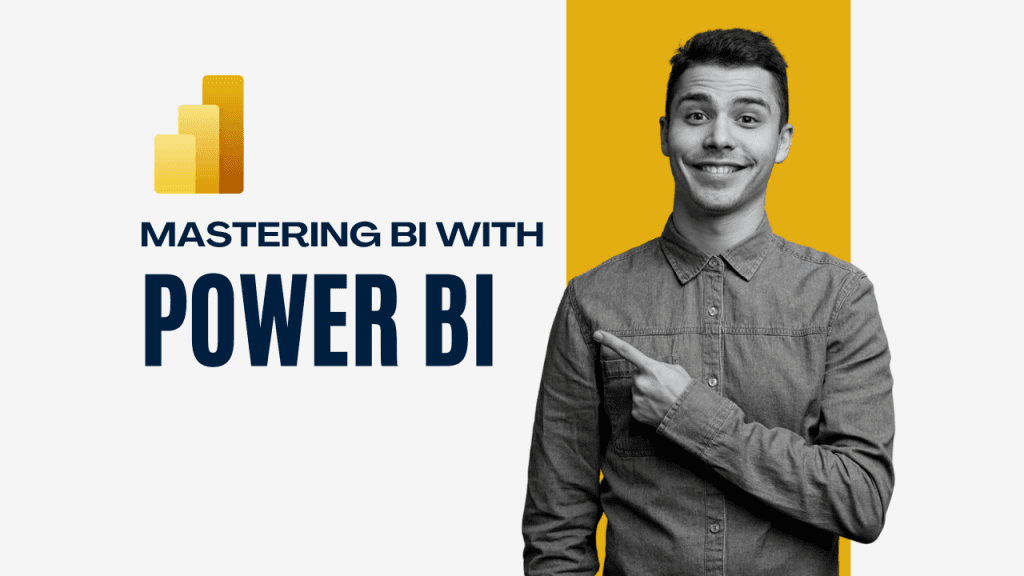
Unlock Your Business Intelligence Potential with Power BI!
6. Comments
Comments improve the readability of the program and make it easier to understand.
- Single-line comments:
// This is a comment
- Multi-line comments:
/* This is a multi-line comment */
Complete Example of the Structure of a Java Program
Here is a complete example to describe the structure of a Java program:
package com.example.myapp; // Package declaration
import java.util.Scanner; // Import statement
public class HelloWorld { // Class declaration
public static void main(String[] args) { // Main method
System.out.println(“Hello, World!”); // Output statement
}
}
Common Java Interview Questions on Program Structure
When preparing for Java 8 programming interview questions or scenario-based interview questions Java, understanding the structure of a Java program is crucial. Below are some commonly asked questions:
Explain the structure of a Java program.
- Be ready to describe each component, including package declarations, imports, and the main method.
Why is the main method static?
- To allow the JVM to call it without creating an object of the class.
What are the key differences between Java 7 and Java 8?
- For example, Java 8 introduced features like Lambda Expressions and the Stream API.
How would you structure a program to handle user input?
- Use classes like
Scanner
for input handling in your program.
- Use classes like
What are some Java 8 coding interview questions for freshers?
- Questions may include default methods in interfaces or the use of functional interfaces
Why is Understanding Program Structure Important?
For freshers preparing for Java interview questions, knowing the program structure:
- Helps in writing organized and efficient code.
- Improves debugging and error tracing.
- Builds a solid foundation for tackling Java 8 coding interview questions.
Unlock Your Business Intelligence Potential with Power BI!
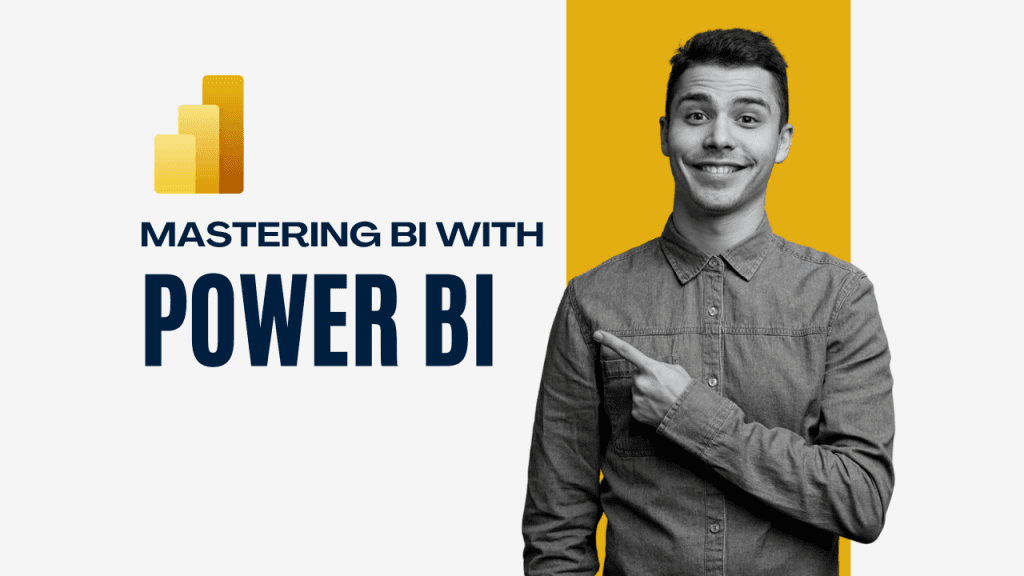
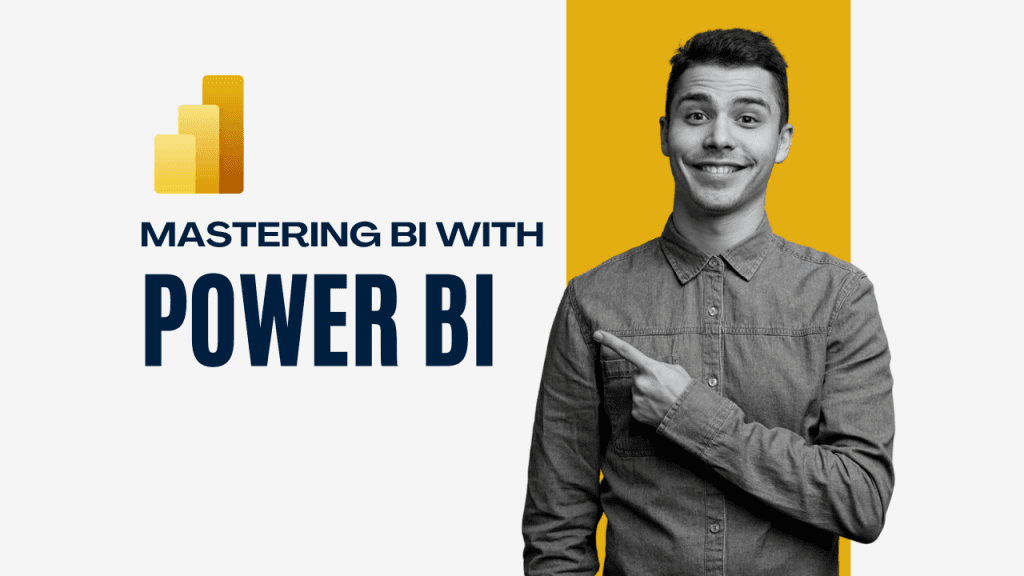