Structure of a Java Program: A Comprehensive Guide
Table of Contents
ToggleJava, a powerful and versatile programming language, is widely embraced for its robust architecture and extensive ecosystem. Understanding the structure of a Java program is fundamental for beginners and professionals aiming to build efficient and scalable applications.
Unlock Your Business Intelligence Potential with Power BI!
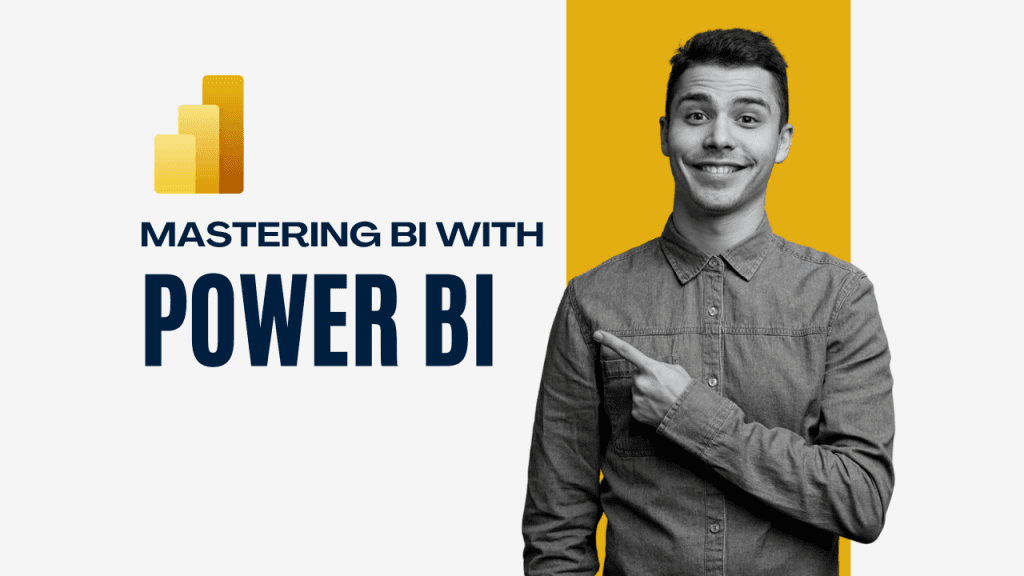
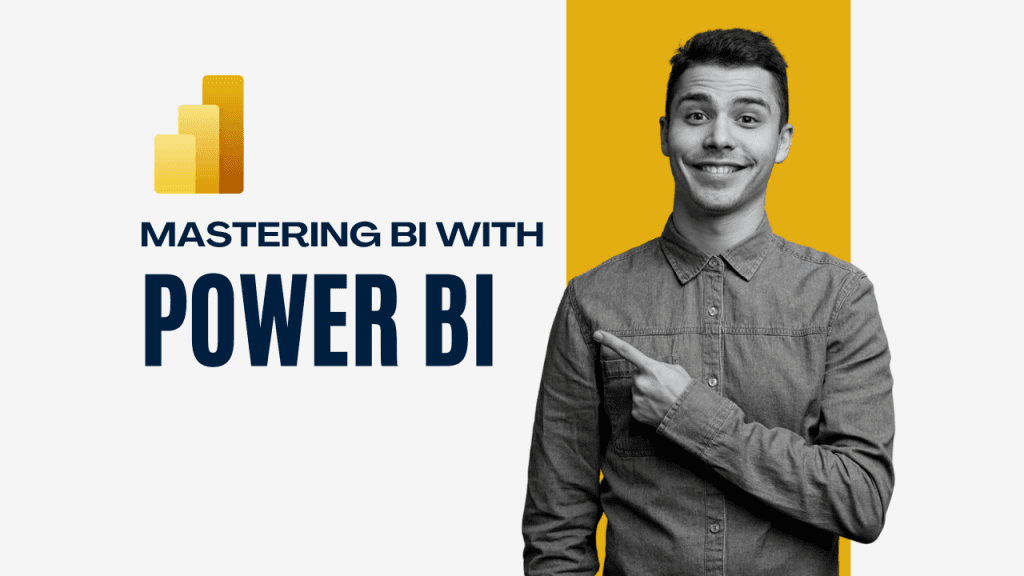
Unlock Your Business Intelligence Potential with Power BI!
Introduction to Java Program Structure
What is a Java Program?
A Java program is a sequence of instructions written in the Java programming language, designed to perform specific tasks. Whether it’s a simple “Hello, World!” or a complex enterprise application, all Java programs follow a predefined structure.
Why Understanding Java Structure is Crucial
The well-defined structure of Java ensures that programs are readable, maintainable, and efficient. It provides a clear blueprint that developers can follow, reducing errors and improving collaboration.
Core Components of a Java Program
Packages and Their Importance
Packages act as containers for classes, helping to organize code logically and avoid name conflicts. Think of packages as folders within a computer directory.
The import
Statement: Bridging Libraries
The import
statement allows you to access predefined classes and methods from Java’s extensive libraries. This enhances functionality without reinventing the wheel.
The Class Declaration
Every Java program revolves around classes. A class is a blueprint that defines the behavior and properties of objects.
The main
Method: Entry Point of Execution
The main
method is the heartbeat of a Java application. It is where the program begins execution, marked by the syntax public static void main(String[] args)
.
Key Syntax Rules in Java
- Case Sensitivity: Java differentiates between
myVariable
andMyVariable
. - Curly Braces: Every block of code must be enclosed in
{}
. - Keywords: Reserved words like
class
,static
, andvoid
cannot be used as identifiers
Unlock Your Business Intelligence Potential with Power BI!
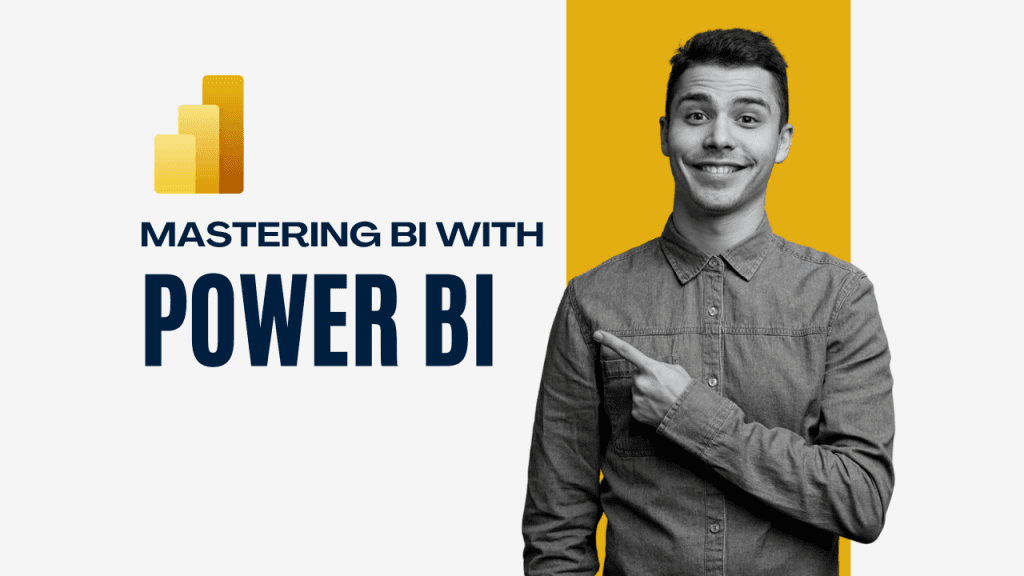
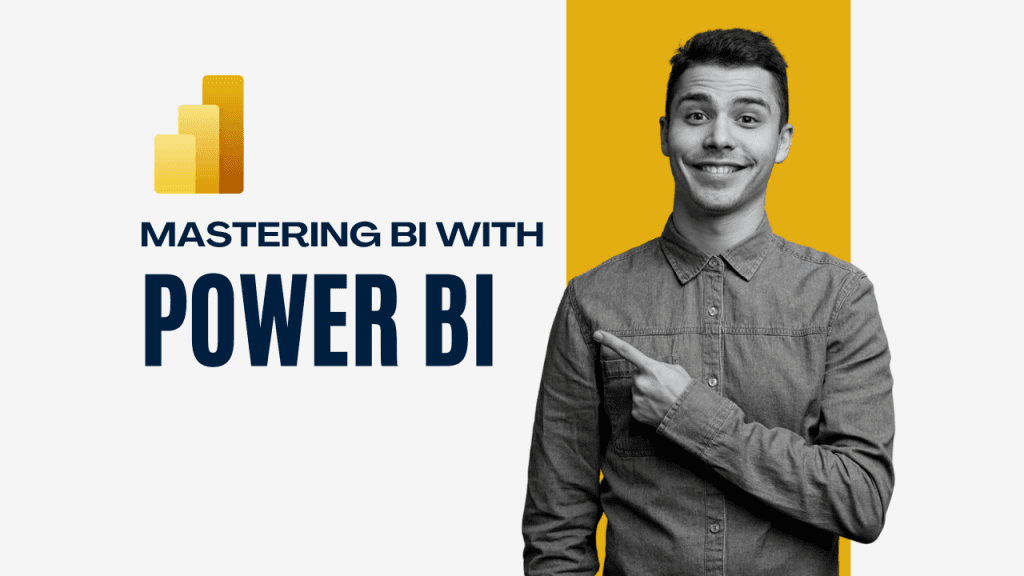
Unlock Your Business Intelligence Potential with Power BI!
Programming Concepts Integrated with Java
Understanding the building blocks of a Java program is crucial for writing effective and efficient code. These components work together to form the foundation of any Java application, from simple scripts to complex software.
1. Variables and Constants
Variables and constants are the primary data holders in a Java program.
- Variables: They are used to store data that may change during program execution. For example:java
int age = 25; // Variable to store age
- Constants: Defined using the
final
keyword, constants store values that remain unchanged throughout the program. For example:javafinal double PI = 3.14159; // A constant for Pi
2. Data Types
Java is a statically-typed language, meaning every variable must have a defined data type. The main types include:
- Primitive Data Types: These include
int
,double
,char
,boolean
, etc. - Reference Data Types: Used for objects like arrays, strings, and user-defined classes.
For example:
javaint number = 10; // Integer data type String message = "Hi"; // Reference data type
3. Classes and Objects
- Classes: The blueprint for creating objects. A class encapsulates data and methods that operate on that data.java
public class Car { String color; void drive() { System.out.println("Car is driving"); } }
- Objects: Instances of a class. They represent the real-world entities in a program.java
Car myCar = new Car(); // Creating an object of Car
4. Methods
Methods are blocks of code designed to perform specific tasks. They help in reusing code and improving modularity.
- Syntax:java
returnType methodName(parameters) { // method body }
- Example:java
public int addNumbers(int a, int b) { return a + b; }
5. Control Flow Statements
Control flow statements determine the order in which instructions are executed. These include:
- Conditional Statements:
if
,else
,switch
- Loops:
for
,while
,do-while
- Example:java
if (age >= 18) { System.out.println("You are an adult."); }
6. Arrays
Arrays store multiple values of the same data type in a single variable.
- Example:java
int[] numbers = {1, 2, 3, 4, 5}; System.out.println(numbers[2]); // Output: 3
7. Packages
Packages organize classes into namespaces, avoiding name conflicts and providing modularity.
- Example:java
package mypackage; import java.util.Scanner;
8. Exception Handling
Java provides robust exception-handling mechanisms to manage runtime errors using
try
,catch
, andfinally
blocks.- Example:java
try { int result = 10 / 0; } catch (ArithmeticException e) { System.out.println("Cannot divide by zero!"); }
9. Input/Output (I/O)
Java’s I/O system handles data input from users and outputs results. It typically uses classes like
Scanner
for input andSystem.out
for output.- Example:java
Scanner scanner = new Scanner(System.in); System.out.print("Enter your name: "); String name = scanner.nextLine(); System.out.println("Hello, " + name);
10. Comments
Comments are non-executable statements used for documentation and code explanation.
- Single-line comment: Starts with
//
- Multi-line comment: Enclosed in
/* */
- Javadoc comment: Begins with
/**
and used for generating documentation
Example:
java// This is a single-line comment /* This is a multi-line comment */ /** * This is a Javadoc comment */
- Variables: They are used to store data that may change during program execution. For example:
Building Blocks of a Java Program
Here’s a step-by-step guide to creating your first Java program:
Open a text editor and write the following code:
javapublic class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Save the file as
HelloWorld.java
.Compile the program using the command:
javac HelloWorld.java
.Run it with:
java HelloWorld
.
Writing a Simple Java Program
Here’s a step-by-step guide to creating your first Java program:
Open a text editor and write the following code:
javapublic class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Save the file as
HelloWorld.java
.Compile the program using the command:
javac HelloWorld.java
.Run it with:
java HelloWorld
.
Best Practices for Writing Java Programs
- Use comments for clarity.
- Follow consistent naming conventions.
- Regularly test and debug your code.
FAQs: Addressing Common Questions on Java Program Structure
What is the role of the
main
method in Java? Themain
method serves as the program’s starting point.How do I structure a large Java application? Break it into multiple packages and classes for modularity.
What are packages, and why are they essential? Packages organize code and prevent class name conflicts.
Can Java programs run without the
main
method? Only special Java programs like applets can bypass themain
method.How can I implement data structures in Java? Use Java’s
java.util
package, which includes classes likeArrayList
andHashMap
.What is the difference between Java and other programming languages? Java is platform-independent, object-oriented, and features automatic garbage collection.
Unlock Your Business Intelligence Potential with Power BI!
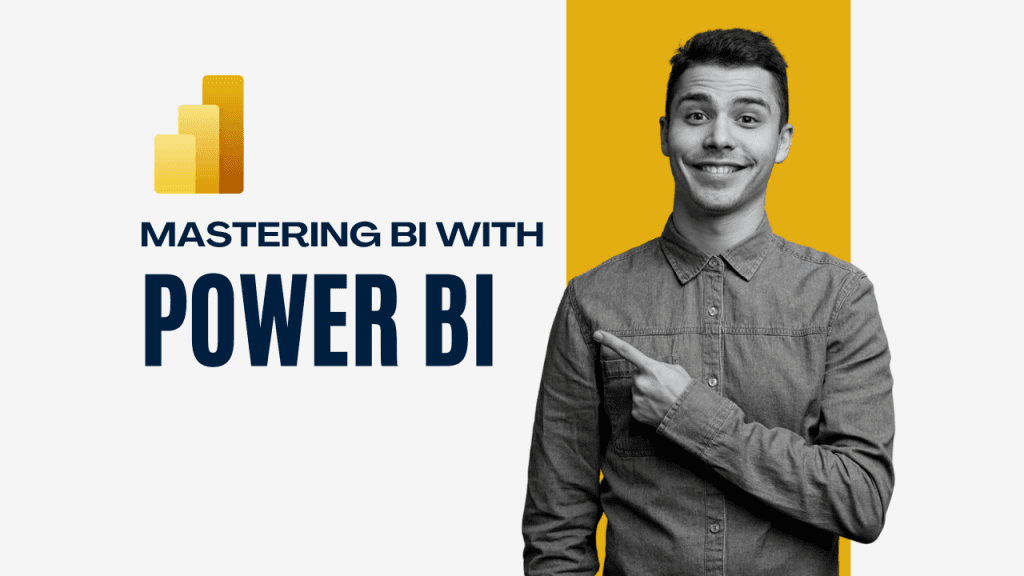
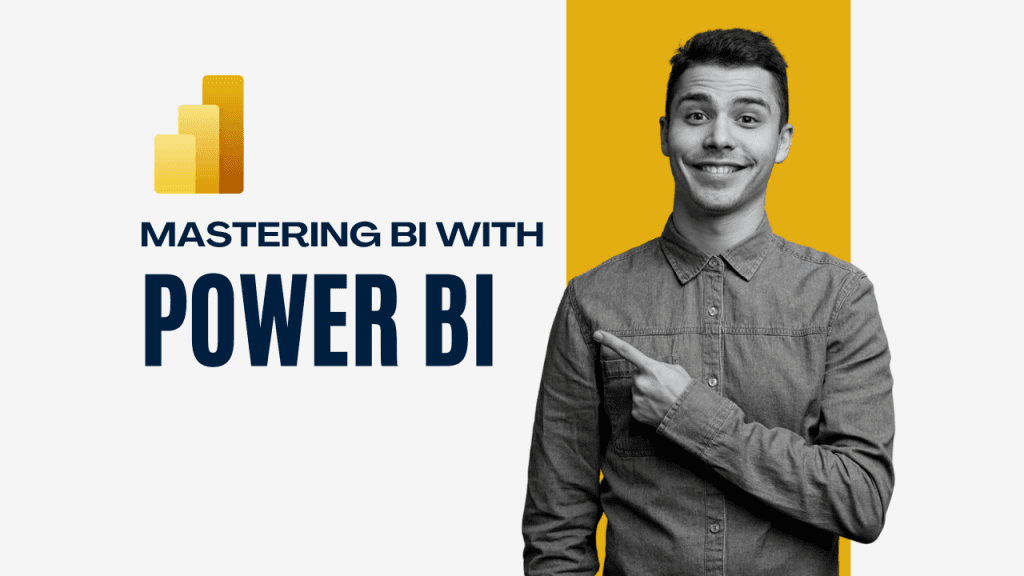