Unlock Your Business Intelligence Potential with Power BI!
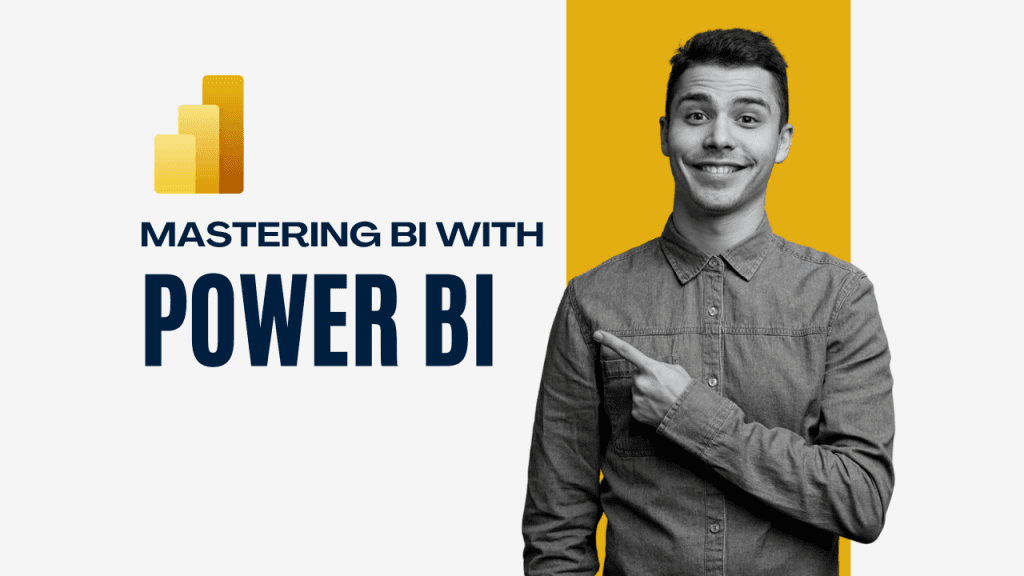
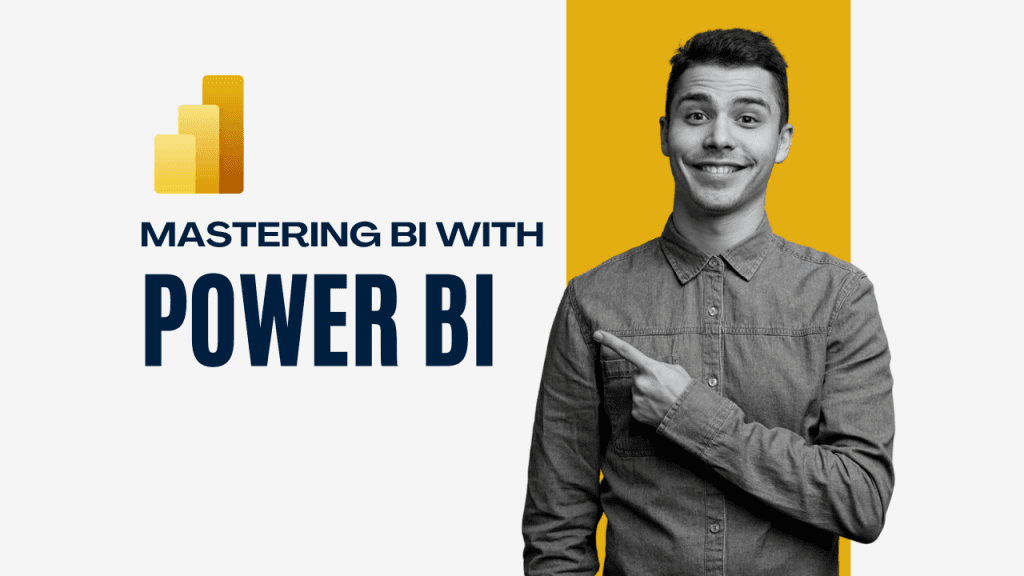
Unlock Your Business Intelligence Potential with Power BI!
Java is a popular and versatile programming language widely used in application development. To write efficient Java programs, it’s essential to understand their structure. In this article, we will delve into the structure of a Java program, its components, and how it is organized. This knowledge is also crucial for answering questions in Java 8 programming interview questions or even scenario-based interview questions in Java.
Table of Contents
ToggleGeneral Structure of a Java Program
The general structure of a Java program consists of multiple components organized in a systematic way. Below are the key components that make up the basic structure of a Java program:
Package Declaration
- A package is a namespace that organizes classes and interfaces.
- Syntax: package mypackage;
Import Statements
- These are used to include external Java classes or packages.
- Example:
- import java.util.Scanner;;
Unlock Your Business Intelligence Potential with Power BI!
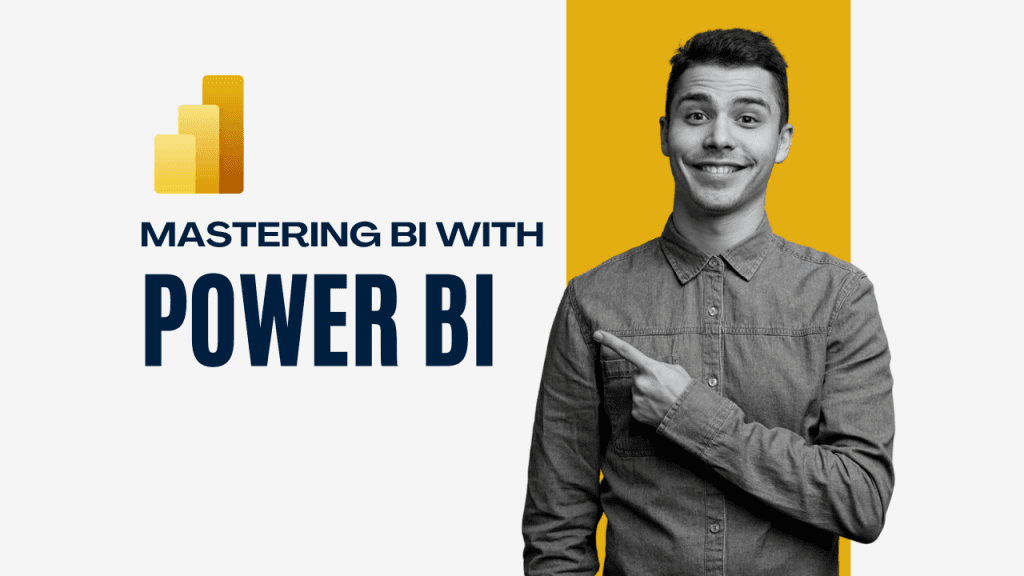
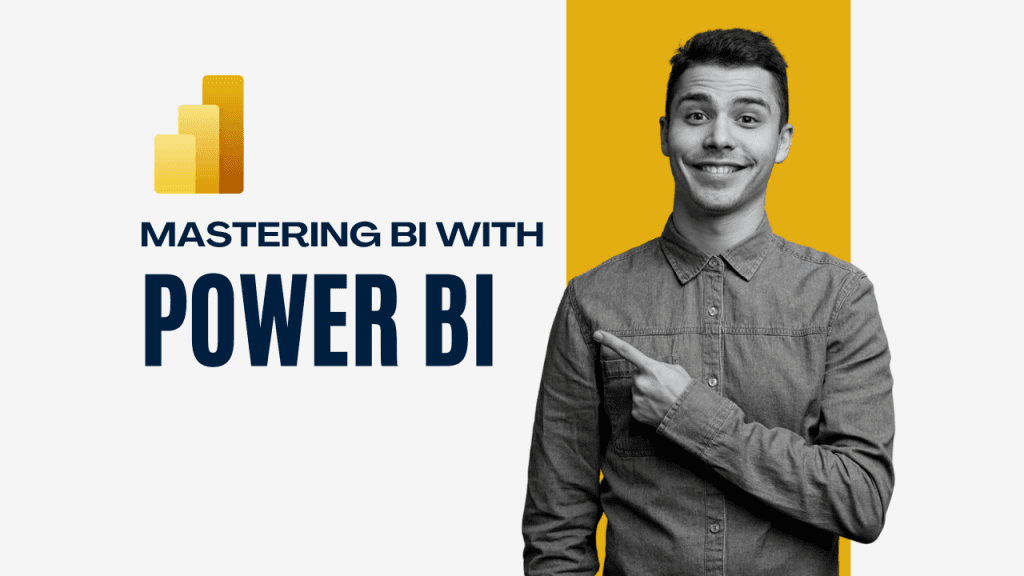
Unlock Your Business Intelligence Potential with Power BI!
Class Declaration
- Every Java program must contain at least one class. It is the fundamental building block.
- Syntax:
- public class MyClass { // Class body }
Main Method
- The
main
method is the entry point of a Java program. - Syntax:
- public static void main(String[] args) { // Code to be executed }
Basic Structure of a Java Program with an Example
- Here is an example to illustrate the structure of a Java program:
// Package declaration
package example;// Import statements
import java.util.Scanner;// Class declaration
public class MyFirstJavaProgram {// Main method
public static void main(String[] args) {
// Statement to print a message
System.out.println(“Welcome to Java Programming!”);
// Example of user input
Scanner scanner = new Scanner(System.in);
System.out.print(“Enter your name: “);
String name = scanner.nextLine();
System.out.println(“Hello, ” + name);
}
}
Explain Structure of a Java Program
- Package Declaration: Organizes your code and avoids name conflicts.
- Import Statements: Allow you to use predefined Java libraries.
- Class Declaration: Encloses the program’s logic and serves as the blueprint for objects.
- Main Method: Acts as the program’s starting point.
- Statements and Expressions: Contain the actual logic, calculations, and operations.
When answering Java 8 coding interview questions, it’s helpful to describe these steps with examples.
Scenario-Based Interview Questions in Java
Understanding the structure of a Java program can also help you tackle scenario-based interview questions in Java. For example:
Question: Describe the use of the
main
method in the structure of a Java program.
Answer: Themain
method serves as the entry point where the Java Virtual Machine (JVM) starts program execution. Without it, the program cannot run.Question: How can you structure a program to read and display user input?
Answer: Use theScanner
class to take input, and place the code inside themain
method or another method.
Java Interview Questions for Freshers
Freshers preparing for interviews might encounter questions such as:
- What is the general structure of a Java program?
- Can you explain the structure of a Java program?
- How do import statements and package declarations fit into the structure of a Java program?
- What is the purpose of the
main
method?
Answering these confidently requires understanding the core components and practicing coding.
Unlock Your Business Intelligence Potential with Power BI!
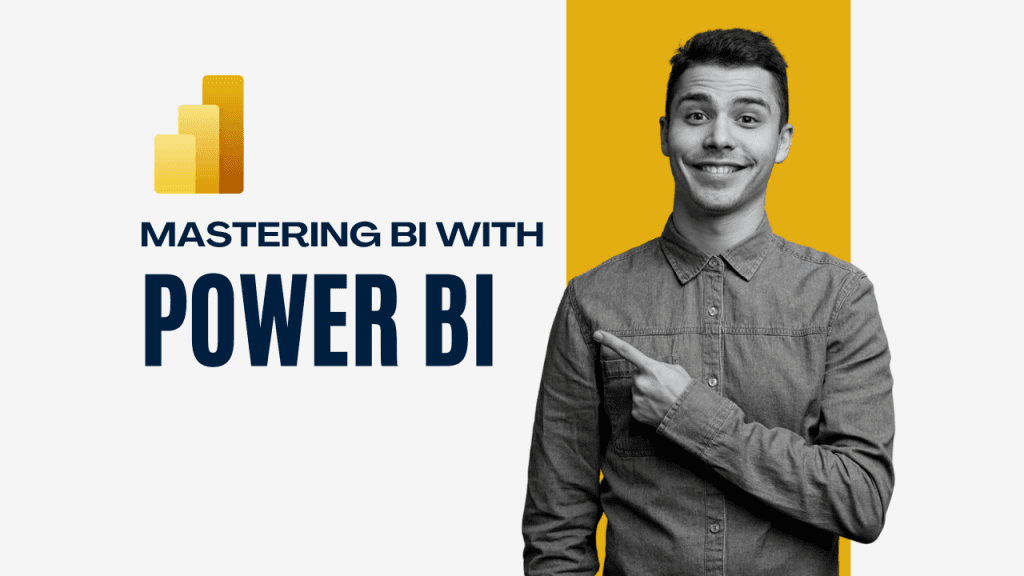
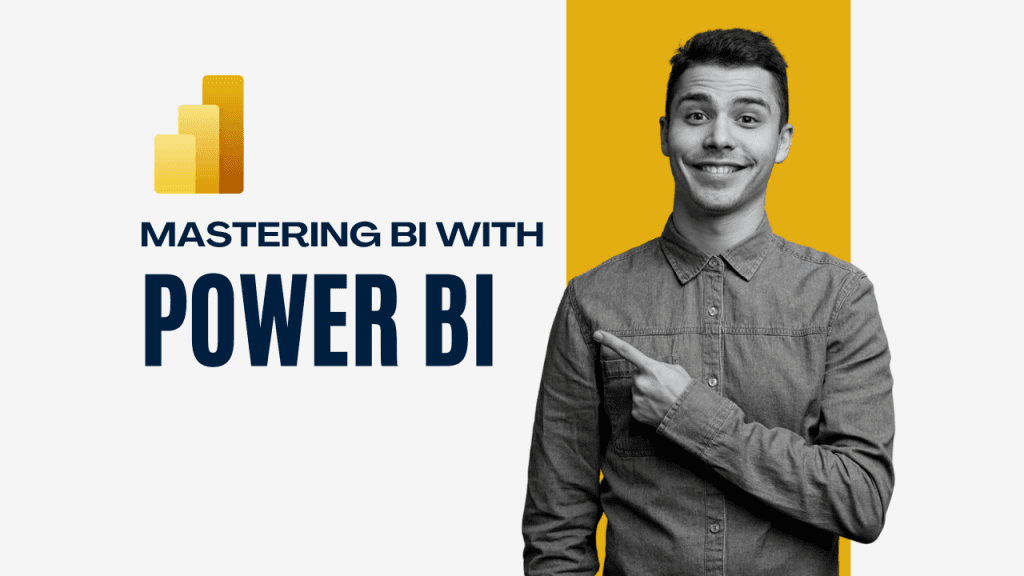