Calculating the factorial of a number is a fundamental concept in programming and plays a significant role in interview preparation. If you’re diving into Java, understanding how to implement a factorial program in Java using a while
loop is essential. This article will walk you through the process step by step, ensuring clarity and mastery.
Factorial Program Using While Loop in Java
ToggleUnlock Your Business Intelligence Potential with Power BI!
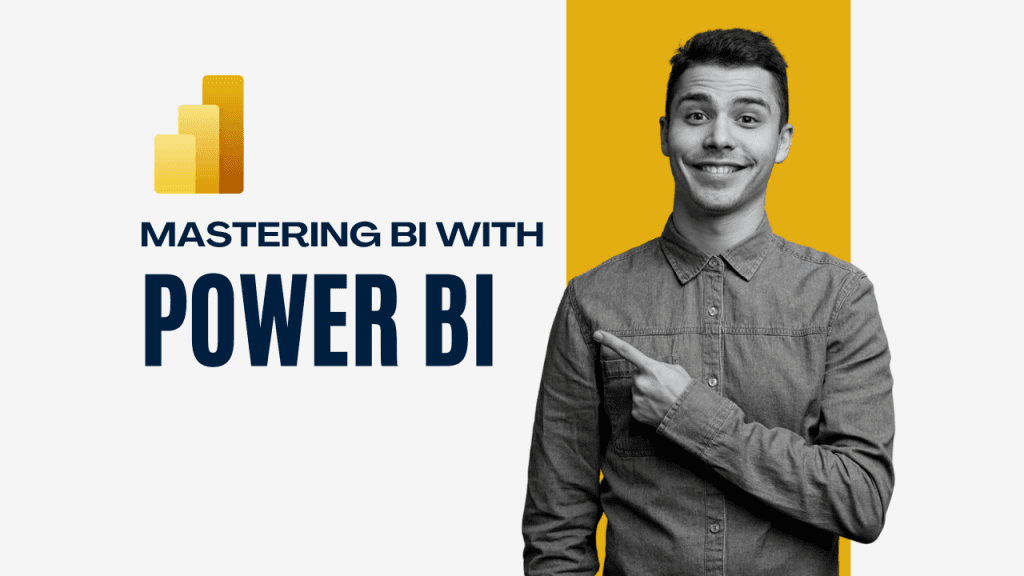
Factorial Program Using While Loop in Java
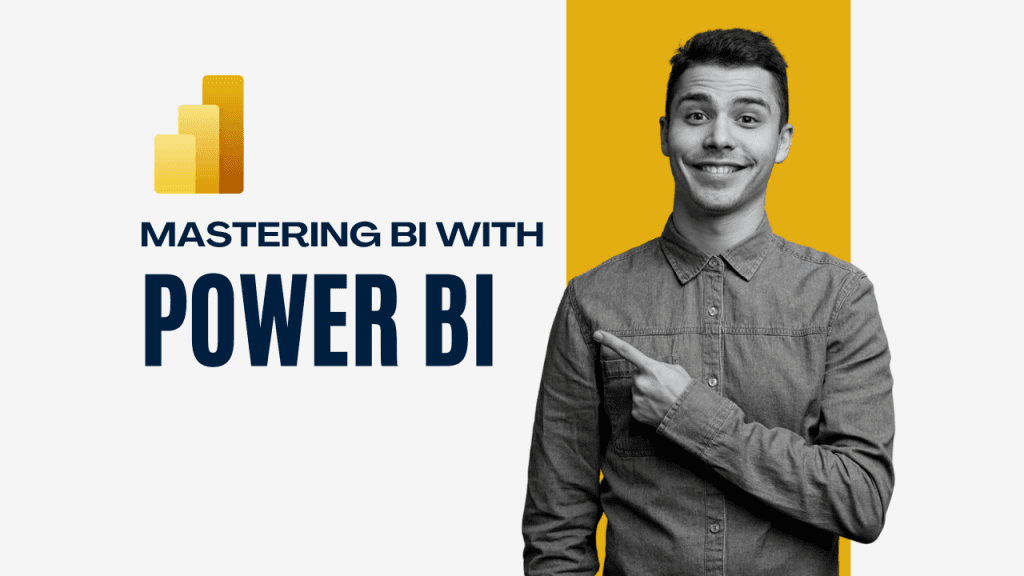
Unlock Your Business Intelligence Potential with Power BI!
What is a Factorial?
A factorial is the product of all positive integers up to a given number. Represented as n!, the factorial of a number is obtained by calculating:
n!=n×(n−1)×(n−2)×…×1n! = n \\times (n-1) \\times (n-2) \\times \\ldots \\times 1n!=n×(n−1)×(n−2)×…×1
For example:
5!=5×4×3×2×1=1205! = 5 \\times 4 \\times 3 \\times 2 \\times 1 = 1205!=5×4×3×2×1=120
0!=10! = 10!=1 (by definition)
Factorials are widely used in mathematics, statistics and programs, this one’s a popular topic for interviews.
Why Use a While Loop?
The while loop is a flexible way to perform an iterative job in Java. A while loop runs while its condition is true, which makes it favorable for jobs like calculating factorials where the number of iterations is dynamically determined.
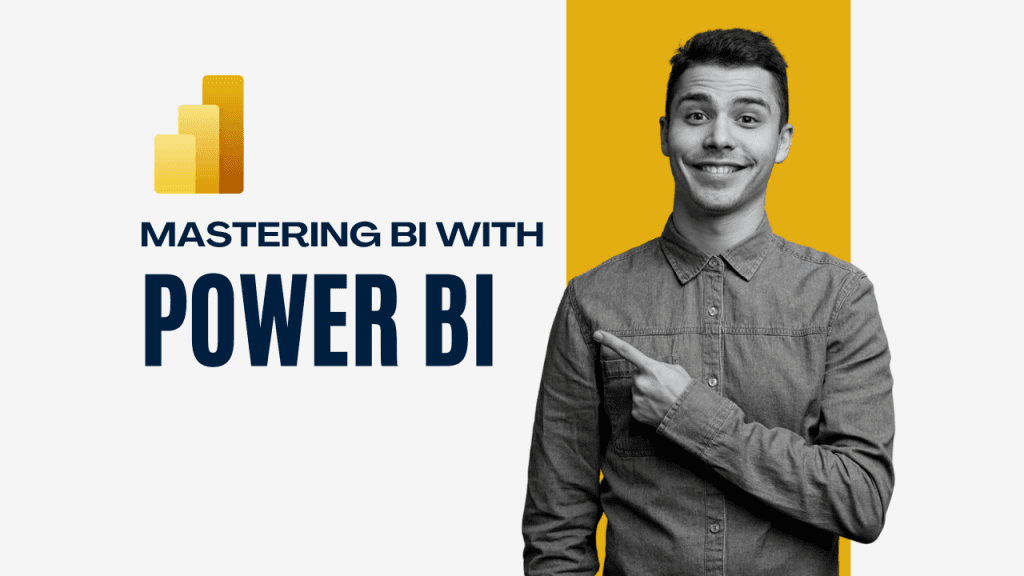
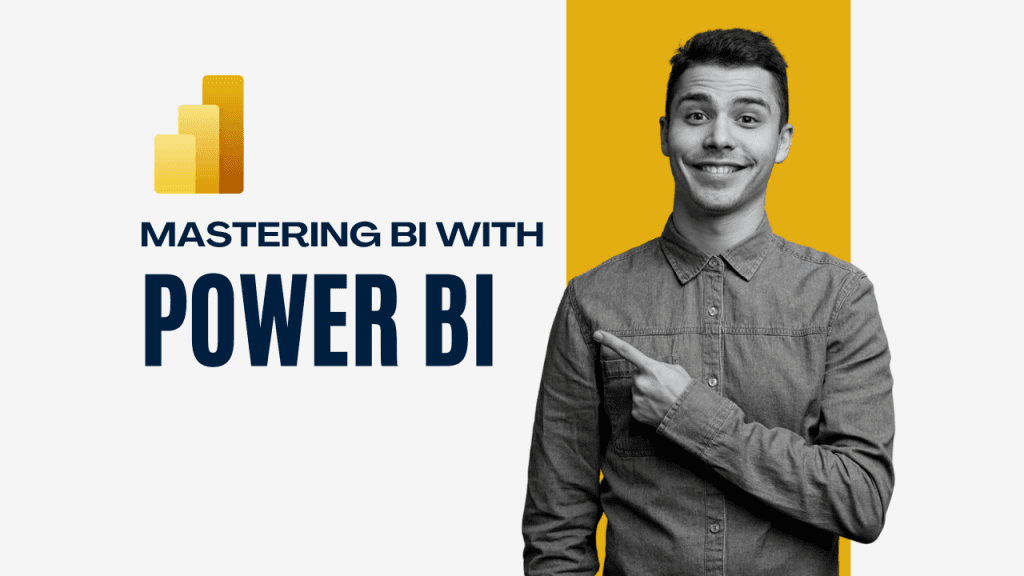
Unlock Your Business Intelligence Potential with Power BI!
Factorial Program in Java Using While Loop
Code Explanation
Here’s a step-by-step breakdown of the factorial program in Java using a while loop:
- Input a Number: The program prompts the user to enter a non-negative integer.
- Initialize Variables: Set up a variable to hold the result (
factorial
) and another for iteration (i
). - Use a While Loop: Multiply
factorial
byi
repeatedly until the loop condition is false. - Display the Result: Output the calculated factorial to the console.
Code implementation
import java.util.Scanner;
public class FactorialWhileLoop {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Input from user
System.out.print(“Enter a non-negative integer: “);
int number = scanner.nextInt();
// Initialize variables
int i = 1;
long factorial = 1; // Use long to handle large results
// Validate input
if (number < 0) {
System.out.println(“Factorial is not defined for negative numbers.”);
} else {
// Calculate factorial using while loop
while (i <= number) {
factorial *= i;
i++;
}
// Output the result
System.out.println(“Factorial of ” + number + ” is: ” + factorial);
}
scanner.close();
}
}
Analyzing the Output
When you run the above program, you’ll see results like:
- Input:
5
- Output:
Factorial of 5 is: 120
This program handles both valid and invalid inputs gracefully.
Common Errors to Avoid
- Forgetting to initialize
factorial
to1
. - Using an incorrect loop condition (
i < number
instead ofi <= number
). - Not accounting for negative input values.
Factorial Using Recursion in Java
- Recursion is another popular way to calculate the factorial of a number in Java. Here’s a quick comparison:
Recursive Implementation
public class FactorialRecursion {
public static long factorial(int n) {
if (n == 0 || n == 1) {
return 1;
}
return n * factorial(n – 1);
}public static void main(String[] args) {
int number = 5;
System.out.println(“Factorial of ” + number + ” is: ” + factorial(number));
}
}
Conclusion
Understanding how to write a factorial program in Java using a while loop is crucial for both beginners and advanced programmers. It builds your logical thinking and problem-solving skills, especially for interview preparation. Whether you use a loop or recursion, the key is to grasp the underlying logic and practice consistently.
FAQs
What is the simplest way to calculate a factorial in Java?
Using a while loop is straightforward for beginners to calculate factorials.How does recursion compare with a while loop for factorial calculation?
Recursion is concise but can lead to stack overflow; loops are more memory-efficient.Can factorial programs handle large numbers in Java?
Yes, by usingBigInteger
, you can manage very large factorials without overflow.Why are factorial programs commonly used in interviews?
They test your understanding of loops, recursion, and edge cases in programming.How can I prepare for interview questions on factorial programs?
Practice implementing factorials using loops and recursion. Understand edge cases and optimize for performance.
Unlock Your Business Intelligence Potential with Power BI!
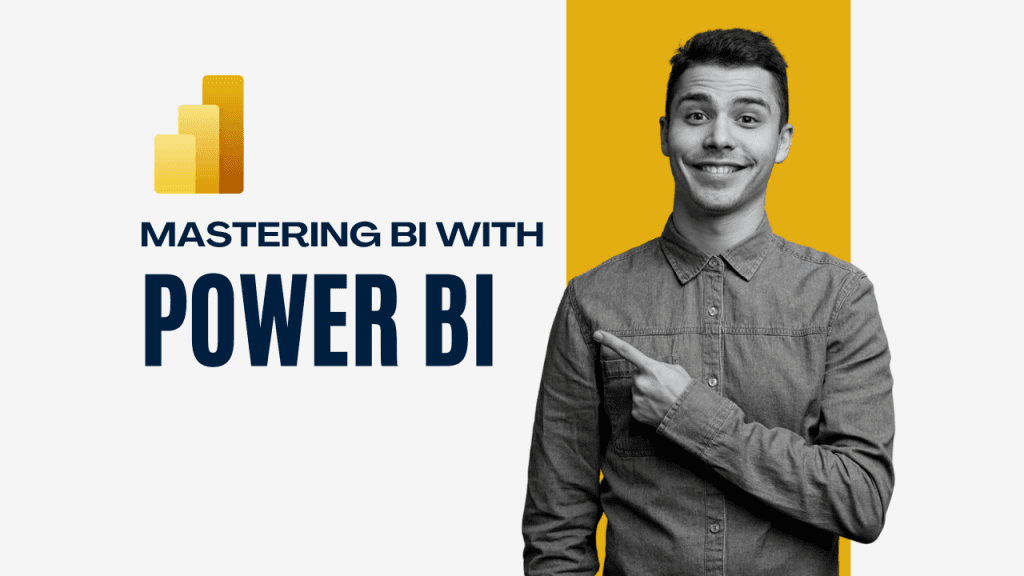
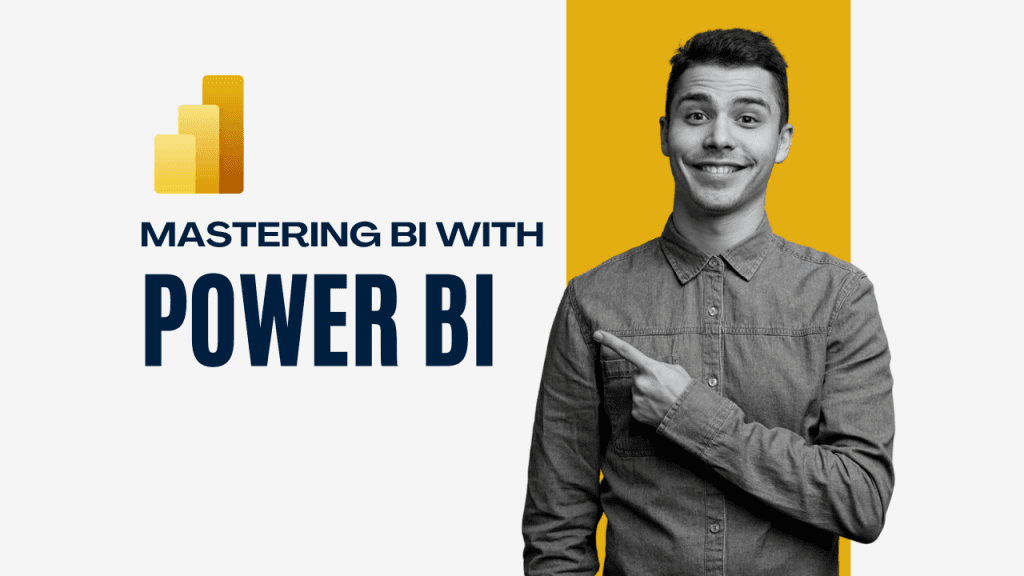