Basic Structure of a Java Program
What is Java?
Java is a popular programming language used to create a wide range of applications, from simple games to complex enterprise systems. It’s known for its simplicity, security, and portability.
Table of Contents
ToggleWhy Java?
- Platform Independence: Java programs can run on any device with a Java Virtual Machine (JVM), making it versatile.
- Object-Oriented: Java follows an object-oriented approach, which helps organize code into reusable components.
- Strong Community: Java has a large and active community, providing extensive support and resources.
- Security: Java has built-in security features to protect against malicious code.
Unlock Your Business Intelligence Potential with Power BI!
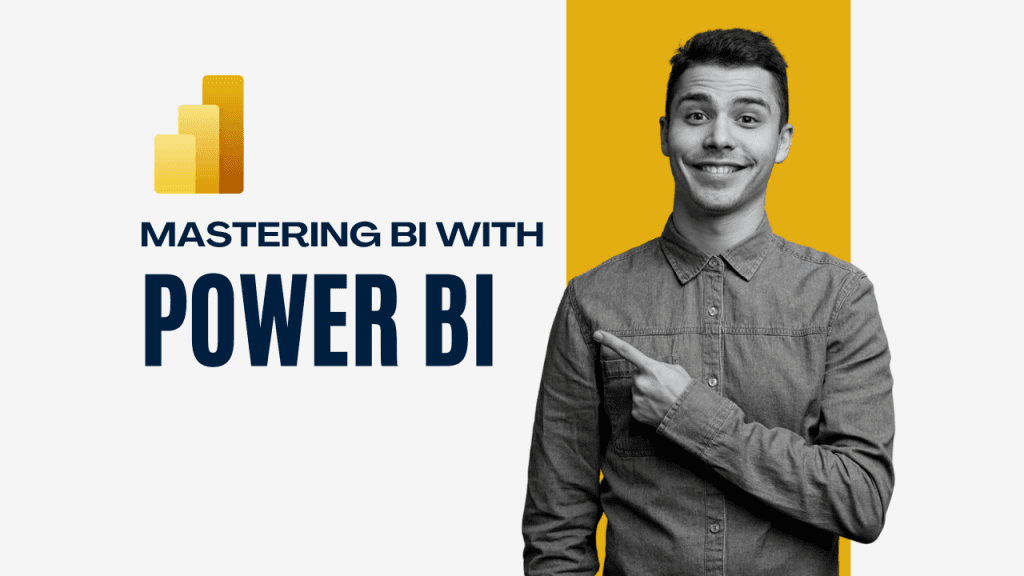
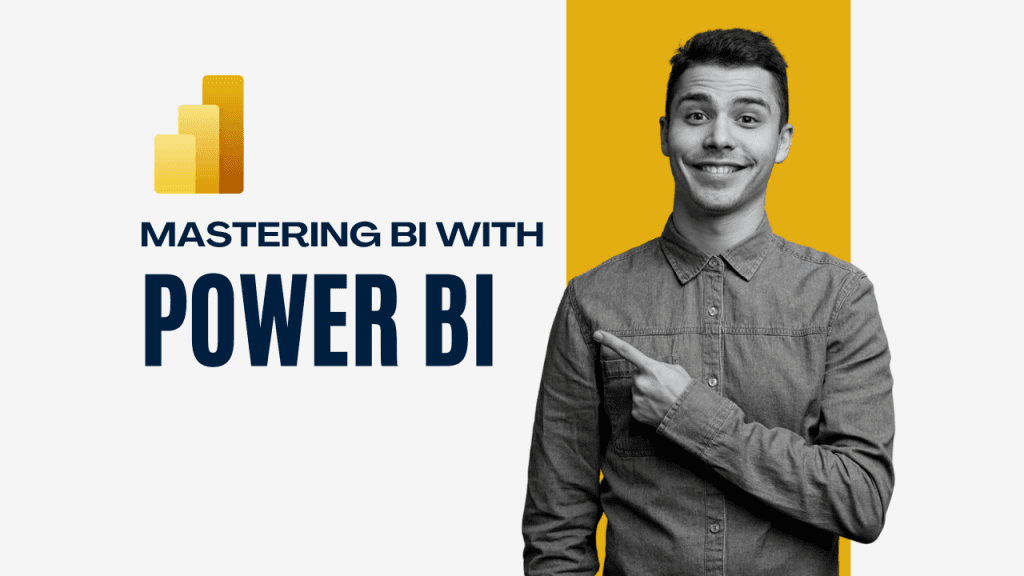
Unlock Your Business Intelligence Potential with Power BI!
Basic Structure of a Java Program
- Every Java program has a specific structure that needs to be followed. Here’s a breakdown of the key components:
Class Declaration
- A class is the basic building block of a Java program. It defines the properties (variables) and behaviors (methods) of objects. The general syntax for a class declaration is:
- public class ClassName {
// Class body
}
public
: This keyword makes the class accessible from anywhere in your program.class
: This keyword indicates that you’re defining a class.ClassName
: This is the name you give to your class.
Main Method
- The
main
method is the entry point of a Java program. It’s where the program execution begins. The syntax for themain
method is:
- Java
public static void main(String[] args) { // Code to be executed }
public
: This keyword makes the method accessible from anywhere in your program.static
: This keyword means the method belongs to the class itself, not to an instance of the class.void
: This indicates that the method doesn’t return any value.main
: This is the specific name of the method that the JVM looks for when running a Java program.String[] args
: This is an array of strings that can be used to pass arguments to the program from the command line.
Unlock Your Business Intelligence Potential with Power BI!
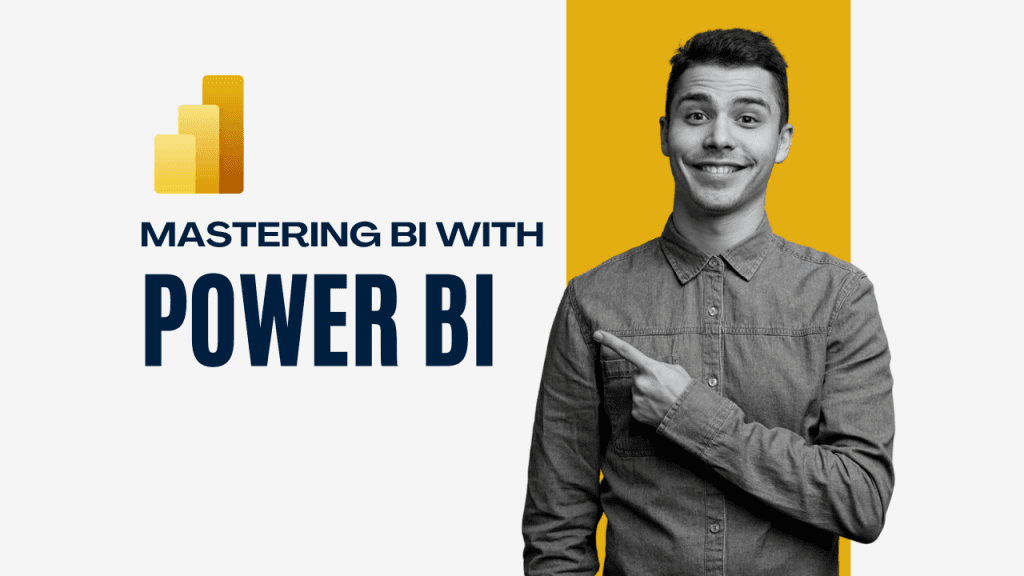
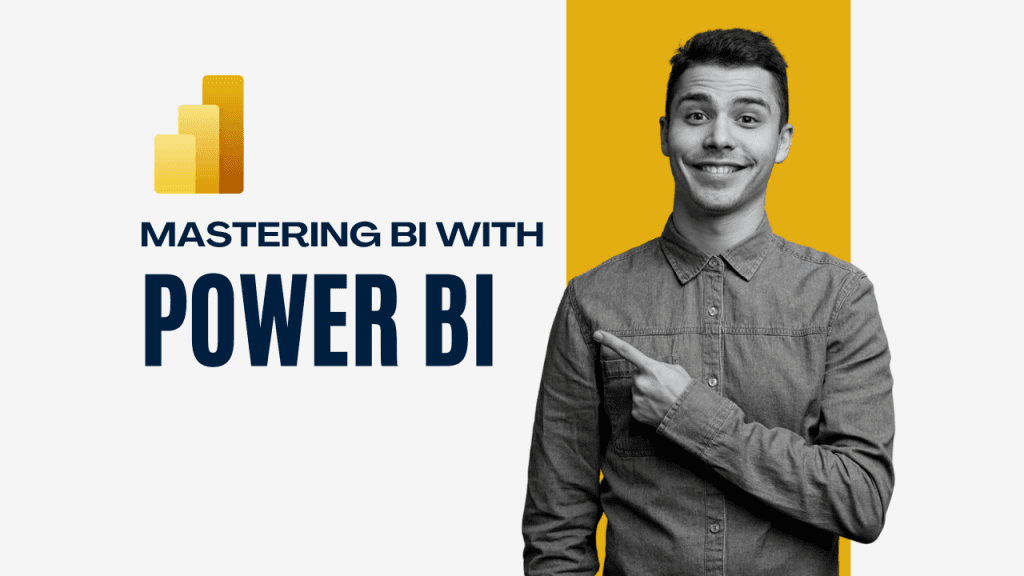
Unlock Your Business Intelligence Potential with Power BI!
Statements
Statements are the instructions that the computer executes. They can be simple or compound. Here are some common types of statements:
- Declaration statements: Used to declare variables.
- Assignment statements: Used to assign values to variables.
- Control flow statements: Used to control the flow of execution (e.g.,
if
,else
,for
,while
). - Method call statements: Used to call methods.
Comments
Comments are used to explain your code and make it easier to understand. They are ignored by the compiler. There are two types of comments in Java:
- Single-line comments: Start with
//
. - Multi-line comments: Start with
/*
and end with*/
.
Example of a Simple Java Program
Here’s a simple Java program that prints “Hello, World!” to the console:
- Single-line comments: Start with
- Java
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello,
World!"); } }
Common Mistakes and Tips
- Missing Semicolons: Every statement in Java must end with a semicolon.
- Case Sensitivity: Java is case-sensitive, so make sure you use the correct capitalization for keywords and variable names.
- Indentation: Indenting your code makes it more readable.
- Use Meaningful Names: Give your variables and methods descriptive names.
- Test Your Code: Write small, testable units of code and test them thoroughly.
Conclusion
Understanding the basic structure of a Java program is crucial for anyone who wants to learn Java programming. By following the guidelines and tips in this article, you can start writing your own Java programs.
FAQs
- What is the difference between a class and an object in Java? A class is a blueprint for creating objects. An object is an instance of a class.
- What is the purpose of the
main
method? Themain
method is the entry point of a Java program. It’s where the program execution begins. - What are variables in Java? Variables are used to store data. They have a data type and a name.
- What are operators in Java? Operators are symbols that perform operations on variables and values.
- What are control flow statements? Control flow statements are used to control the flow of execution of a program.
Unlock Your Business Intelligence Potential with Power BI!
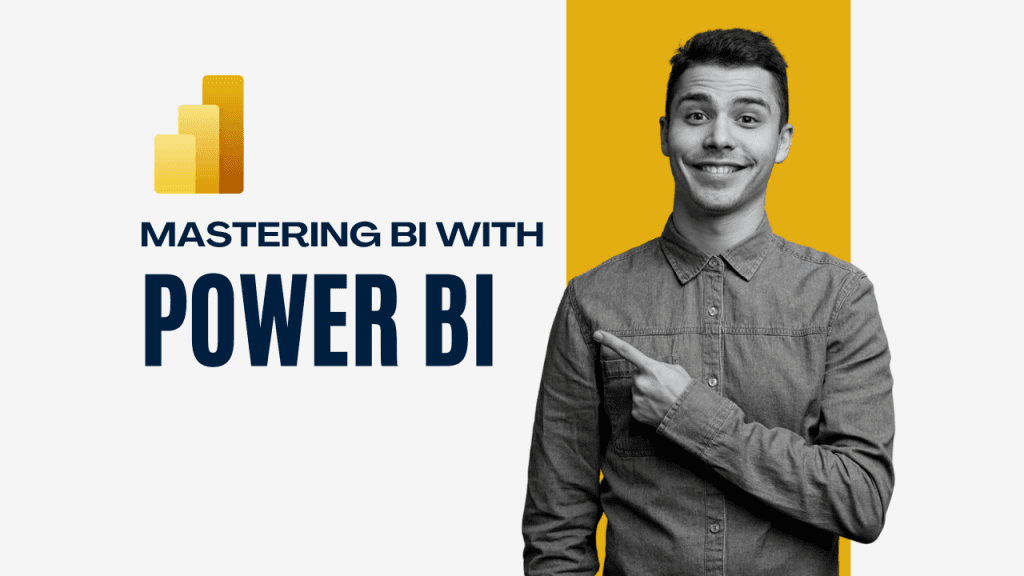
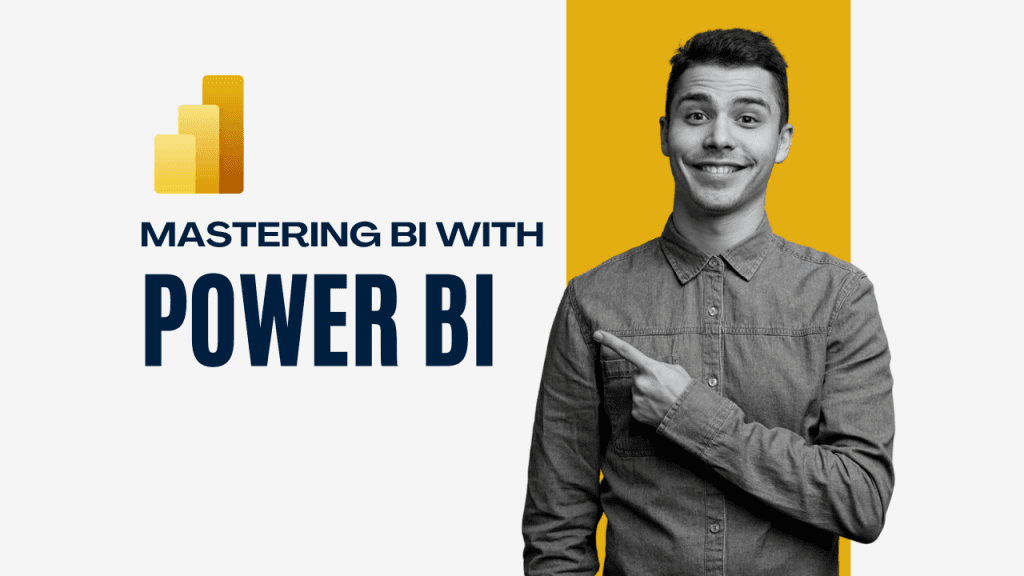