Java is one of the most popular programming languages, and understanding its structure is essential for both beginners and experienced developers. The structure of a Java program lays the foundation for creating well-organized, readable, and maintainable code. In this article, we will explore and explain the structure of a Java program step by step.
Table of Contents
ToggleBasic Structure of a Java Program
The structure of a Java program consists of several essential components, arranged in a specific order to ensure proper functioning. Here’s a breakdown of the key components:
Unlock Your Business Intelligence Potential with Power BI!
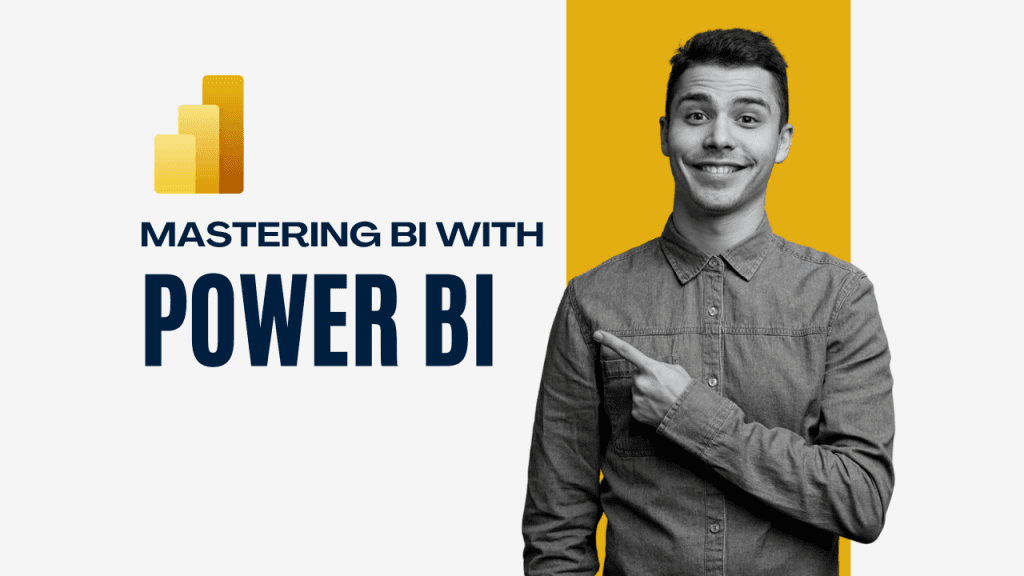
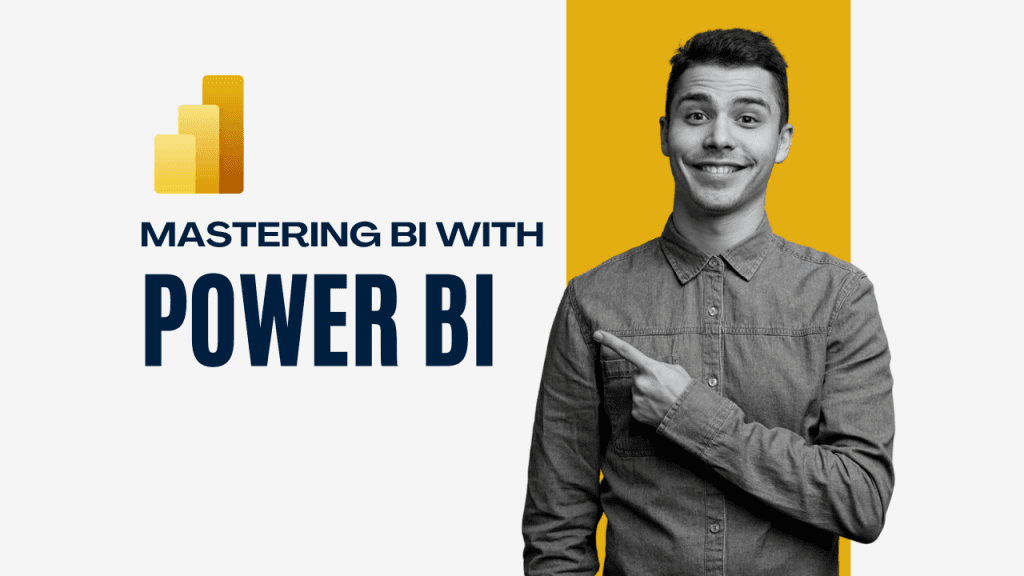
Unlock Your Business Intelligence Potential with Power BI!
Documentation Section
This section contains comments to describe the program. Comments are not executed by the compiler but help developers understand the purpose of the code.
Example:
// This is a single-line comment /*
This is a multi-line comment */
Package Declaration (Optional)
If the program belongs to a package, you declare it at the top. Packages help organize classes and interfaces.
Example:
package com.example.myapp;
Import Statements
These allow you to include other classes or packages required in the program.
Example:
import java.util.Scanner;
Class Definition
A Java program must have at least one class. The class name should match the file name.
Example:
public class HelloWorld { }
Main Method
This is the entry point of a Java program where the execution begins.
Example:
public static void main(String[] args) { System.out.println(“Hello, World!”); }
Detailed Explanation of Java Program Structure
Class Declaration
Every Java program is built around classes. A class is defined using the class
keyword, and all the code resides within its curly braces {}
.
public class MyProgram { // Class content goes here }
The Main Method
The main method is the heart of any Java application. It uses the following signature:
public static void main(String[] args) {
}
public
makes it accessible from anywhere.static
allows the method to be called without creating an object.void
indicates no return value.String[] args
accepts command-line arguments.
Unlock Your Business Intelligence Potential with Power BI!
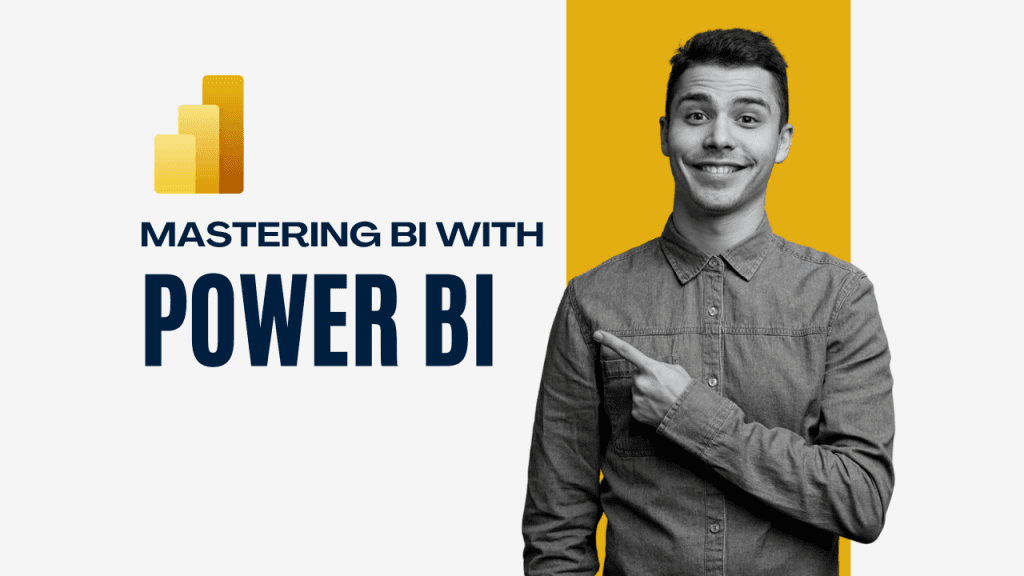
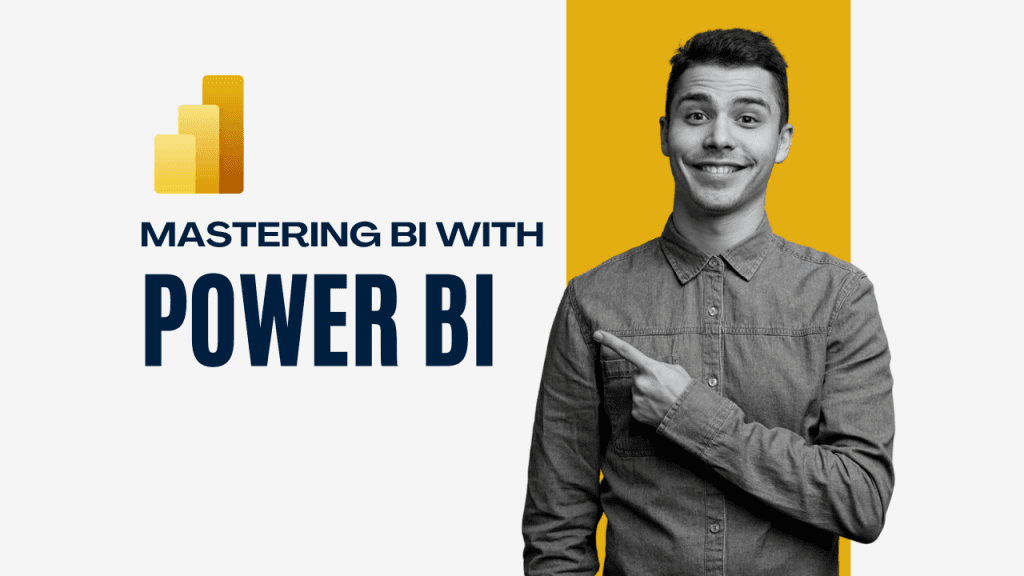
Unlock Your Business Intelligence Potential with Power BI!
Statements
Statements inside the main
method perform the actual tasks of the program.
public static void main(String[] args)
System.out.println(“Welcome to Java Programming!”);
Execution Flow
The program starts execution from the main
method and runs the statements sequentially. If there are methods or functions, they are called explicitly.
Common Questions on the Structure of Java Program
Understanding the structure of Java program is vital for acing technical interviews. Here are some common Java interview questions:
Explain the structure of Java program.
Interviewers often ask candidates to describe the structure of a Java program and explain its components in detail.Scenario-based interview questions in Java.
Questions like “What happens if the main method is not declared static?” or “How do import statements work in Java?” test practical understanding.Java 8 coding interview questions.
Java 8 introduced features like lambdas and streams. Knowing how to incorporate these within the program structure is key. For instance, understanding how to use lambda expressions to simplify code can make a significant difference.Java interview questions for freshers.
Freshers should prepare to explain the structure of Java program and provide examples of well-structured code during interviews.
Tips for Beginners
- Always start your program with a clear structure, including comments to explain the purpose of each section.
- Learn how to structure Java programs by practicing small examples before moving to complex projects.
- Familiarize yourself with scenario-based questions to better understand real-world applications of Java structure.
Unlock Your Business Intelligence Potential with Power BI!
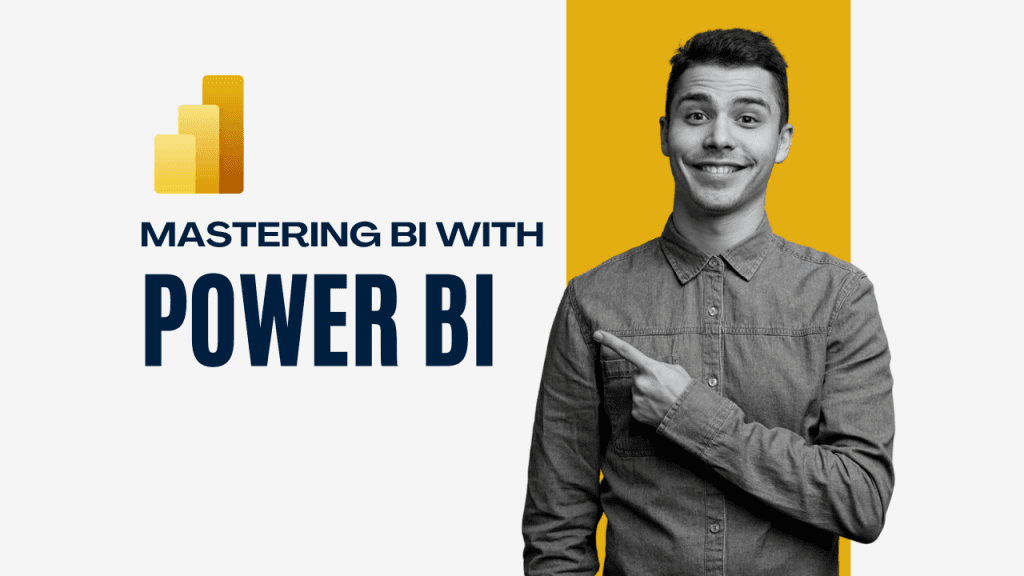
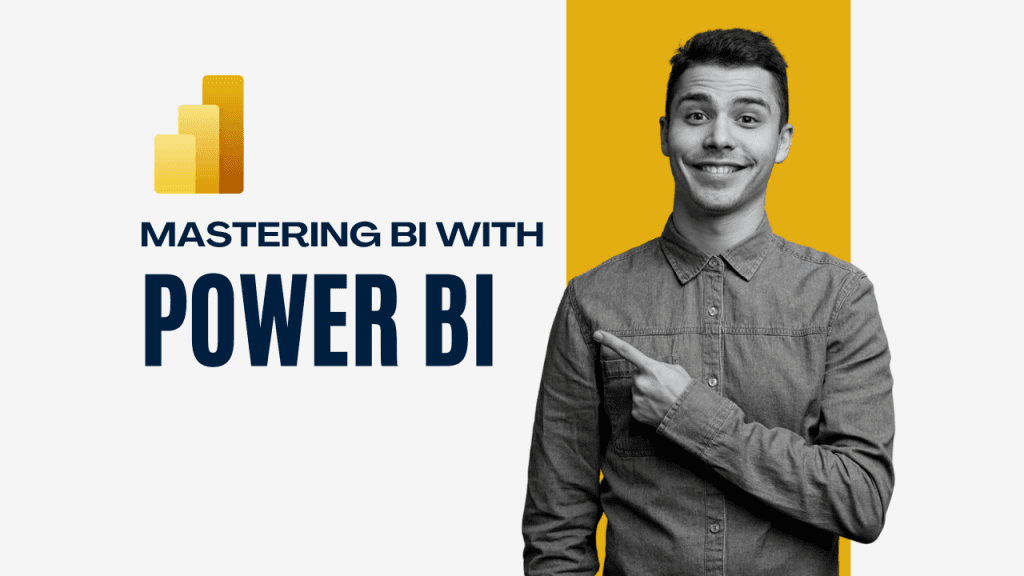