Preparing for a Laravel interview can be challenging, especially when you’re aiming to stand out among other candidates. To ensure success, it’s essential to familiarize yourself with the Laravel interview questions, key concepts, and the framework’s core functionalities. This guide provides a curated list of the most important interview questions for Laravel, along with detailed answers to help you ace your next technical round.
Top Laravel Interview Question
Table of Contents
Toggle1. What is Laravel?
Laravel is an open-source PHP framework that follows the Model-View-Controller (MVC) architecture. It simplifies common tasks such as routing, sessions, caching, and authentication, making it a favorite among developers. Understanding the basics is crucial, as it often comes up in questions in the interview.
Unlock Your Business Intelligence Potential with Power BI!
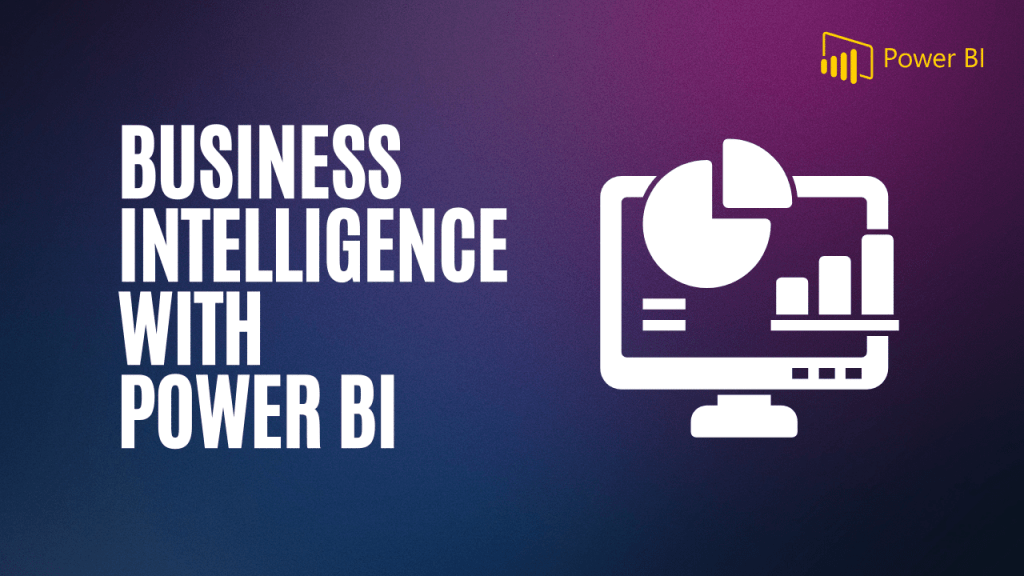
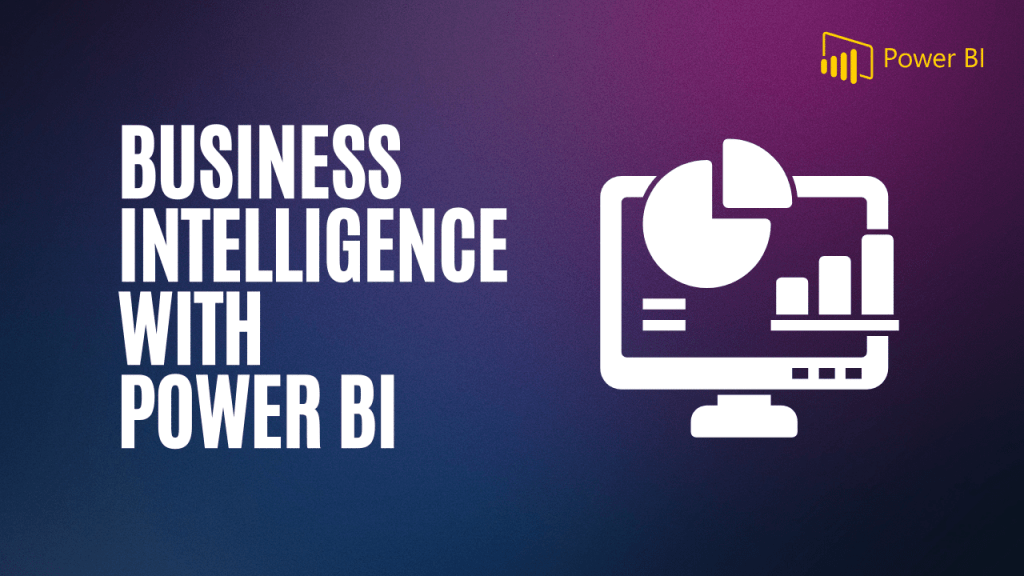
Unlock Your Business Intelligence Potential with Power BI!
2. Why is Laravel Popular Among Developers?
Laravel’s elegant syntax, robust features, and extensive ecosystem make it popular. Features like Eloquent ORM, Blade templating, and a vast library of packages contribute to its dominance. Many php Laravel interview questions revolve around these features.
3. What is the MVC Architecture in Laravel?
Laravel’s MVC (Model-View-Controller) architecture separates the application’s logic, UI, and data. This structure improves code maintainability and scalability. Expect questions for PHP interview that test your understanding of MVC principles.
4. Explain Routing in Laravel.
Routing in Laravel allows developers to define application URLs and their associated logic. Routes can be grouped, named, and secured. Many interview questions for Laravel will require you to demonstrate routing knowledge with examples.
Basic Routing: Laravel routes can be as simple as specifying the URL and corresponding action in the web.php
file. For example:
Route::get('/home', [HomeController::class, 'index']);
Named Routes: Named routes make it easier to link to specific routes by name rather than hardcoding URLs, which is useful for managing large applications.
Route Groups: Grouping routes can help organize and apply shared attributes, such as middleware, to several routes at once, helping to improve readability and maintainability
5. What is Eloquent ORM?
Eloquent is Laravel’s Object-Relational Mapping (ORM) tool. It simplifies database operations and allows developers to interact with the database using models. Be prepared to answer php Laravel interview questions about Eloquent relationships, such as one-to-one, one-to-many, and many-to-many.
6. How Does Laravel Handle Middleware?
Middleware in Laravel acts as a filter for HTTP requests. It allows you to verify user authentication, log activities, and more. You may encounter questions in the interview that ask for examples of custom middleware.
7. What is Blade Templating?
Blade is Laravel’s templating engine that supports data display, loops, and conditionals directly in your view files. Laravel interview questions often include tasks like creating a simple Blade template for dynamic content rendering.
8. How Do You Use Artisan Commands in Laravel?
Artisan is Laravel’s built-in command-line interface, used for creating models, controllers, migrations, and more. Mastering Artisan commands is vital, as they’re frequently mentioned in interview questions for Laravel.
Unlock Your Business Intelligence Potential with Power BI!
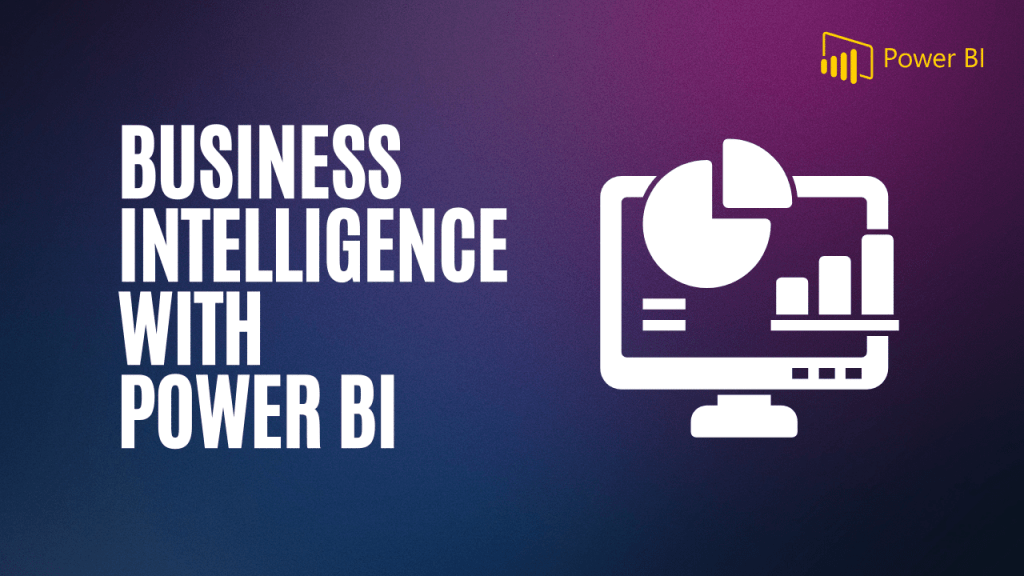
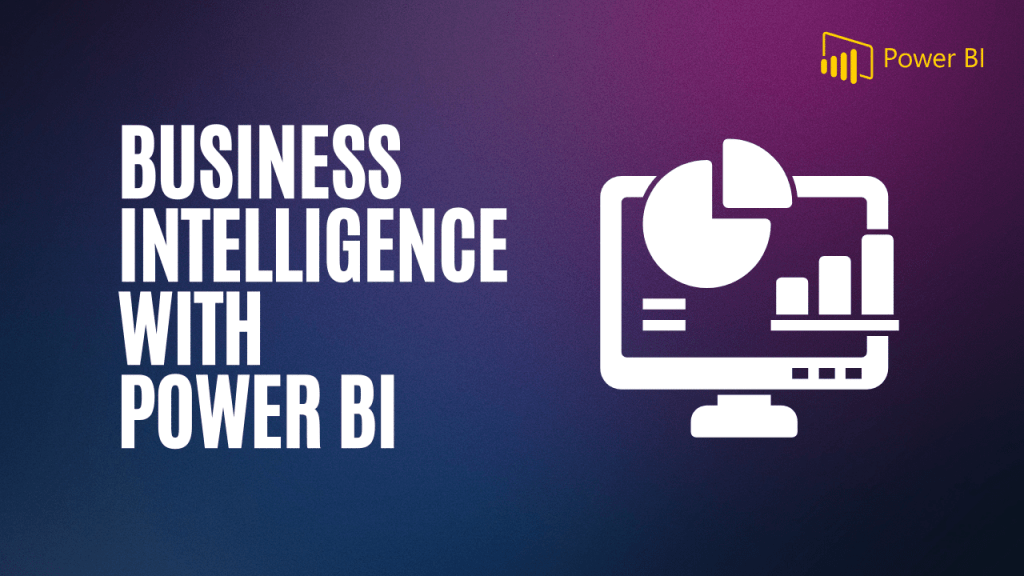
Unlock Your Business Intelligence Potential with Power BI!
9. What is a Service Provider in Laravel?
Service providers are the central place for configuring application services. They are essential for registering bindings in the service container. Be ready to answer questions for PHP interview related to service providers and dependency injection.
10. How Does Laravel Handle Authentication?
Laravel simplifies authentication with pre-built login, registration, and password reset functionalities. Advanced Laravel interview questions may explore custom authentication systems or API authentication using Laravel Passport.
11. What is Laravel Migration?
Migrations in Laravel act as version control for your database, allowing you to modify tables and columns easily. Many php Laravel interview questions involve writing a sample migration or explaining rollback mechanisms.
12. Explain Laravel’s Event-Listener Mechanism.
Laravel’s event system provides a way to decouple various parts of your application. Questions like these are common in interview question and answer discussions.
13. How Do You Implement Task Scheduling in Laravel?
Using Laravel’s Task Scheduler, developers can automate cron jobs through Artisan commands. Expect questions in the interview about real-world scenarios where task scheduling is applicable.
14. What is CSRF Protection in Laravel?
Laravel automatically protects your application from cross-site request forgery (CSRF) attacks by generating a CSRF token for each session. Many interview questions for Laravel focus on security practices like CSRF protection.
15. How Do You Debug in Laravel?
Laravel provides robust debugging tools, including error logs, debug mode, and the Laravel Debugbar. Understanding these tools is crucial for answering questions for PHP interview confidently.
Frequently Asked Questions
1. What Are Common Topics for Laravel Interviews?
Topics include MVC architecture, routing, Eloquent ORM, Blade templating, and middleware. Most php Laravel interview questions focus on these areas.
2. How Should I Prepare for a Laravel Interview?
Study common Laravel interview questions, practice coding, and review Laravel’s official documentation.
3. What is the Difference Between Laravel and Other PHP Frameworks?
Laravel stands out for its elegant syntax, robust tools, and extensive package library. Questions like these are typical in interview question and answer sessions.
4. Can I Expect Coding Challenges During Laravel Interviews?
Yes, coding challenges based on interview questions for Laravel are common, so practice implementing key Laravel features.
5. Are There Advanced Laravel Interview Topics?
Advanced topics include API development, custom authentication, and real-time broadcasting. These often feature in questions in the interview for experienced developers.
Unlock Your Business Intelligence Potential with Power BI!
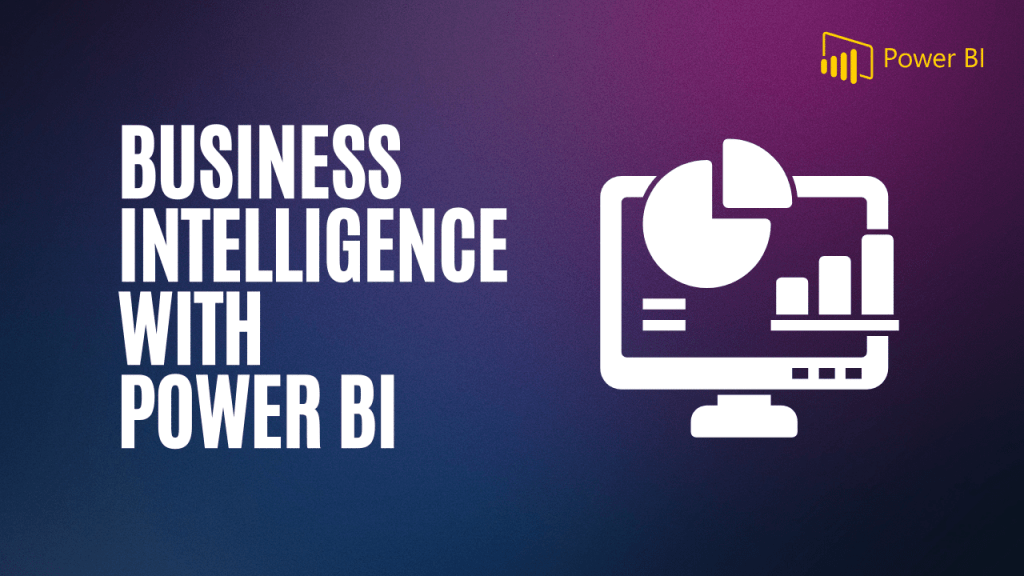
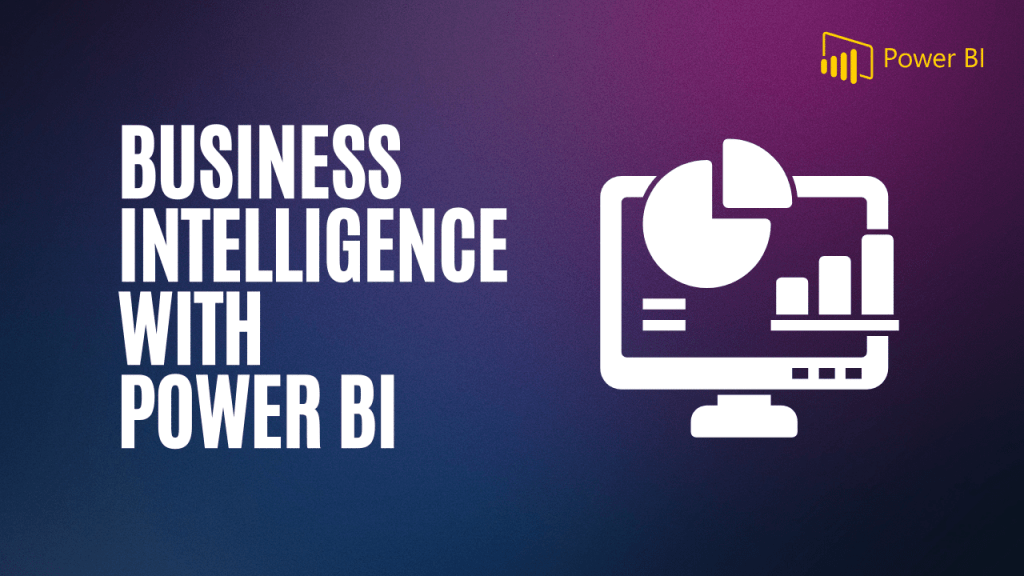